 |
CS 635: Advanced Object-Oriented Design & Programming |
|
---|
Spring Semester, 1998
Design Patterns Intro
To Lecture Notes Index
San Diego State University -- This page last updated 11-Feb-98
Contents of Doc 4, Design Patterns Intro
...References | slide # 1 |
...Design Patterns | slide # 2 |
......OMT-Based Notation | slide # 4 |
......Design Principle 1 | slide # 8 |
......Design Principle 2 | slide # 10 |
......Parameterized Types | slide # 11 |
......Designing for Change | slide # 12 |
References
Design Patterns: Elements of Resuable Object-Oriented Software, Gamma, Helm,
Johnson, Vlissides, Addison-Wesley, 1995
Design Patterns
What is a Pattern?
- "Each pattern describes a problem which occurs over and
over again in our environment, and then describes the
core of the solution to that problem, in such a way that you
can use this solution a million times over, without ever
doing it the same way twice"
- Christopher Alexander
A pattern has four essential elements
Pattern name
Problem
Solution
- "the pattern provides an abstract description of a design
problem and a general arrangement of elements solves it"
Consequences
| | Purpose | |
Scope | Creational | Structural | Behavioral |
Class | Factory Method | Adapter | Interpreter
Template Method |
Object | Abstract factory
Builder
Prototype
Singleton | Adapter
Bridge
Composite
Facade
Flyweight
Proxy | Chain of
Responsibility
Command
Iterator
Mediator
Memento
State
Strategy
Visitor
|
OMT-Based Notation Examples from Text
Class Diagram
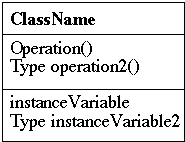
Object Diagram
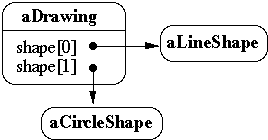
Inheritance
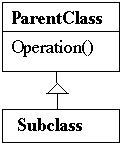
OMT-Based Notation Examples from Text
Abstract and Implementation
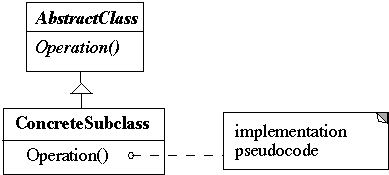
Class Relations
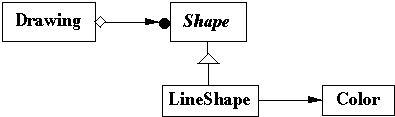
Creating

OMT-Based Notation Examples from Text
Object Interaction
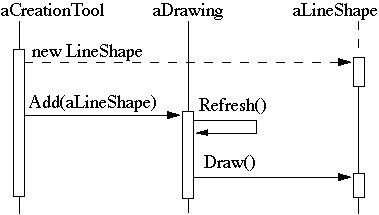
Object, Classes and Type (Again)
Signature
- An operations name, parameters, and return type
Interface
- Set of all signatures defined by an object's operations
Type
- A name used to denote a particular interface
Objects may have many types
Different objects in share a type
Subtype
- A type is a subtype of another it its interface contains the
interface of its supertype
Class
- A class defines an object's implementation
Design Principle 1
Program to an interface,
not an implementation
Use abstract classes (and/or interfaces in Java) to define
common interfaces for a set of classes
Declare variables to be instances of the abstract class not
instances of particular classes
Benefits of programming to an interface
- Client classes/objects remain unaware of the classes of
objects they use, as long as the objects adhere to the
interface the client expects
- Client classes/objects remain unaware of the classes
that implement these objects. Clients only know about the
abstract classes (or interfaces) that define the interface.
Programming to an Interface - Example
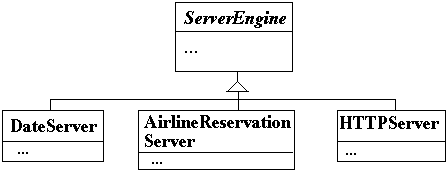
class A
{
DateServer myServer;
public operation()
{ myServer.someOp(); }
}
class B
{
ServerEngine myServer;
public operation()
{ myServer.someOp(); }
}
Design Principle 2
Favor object composition
over class inheritance
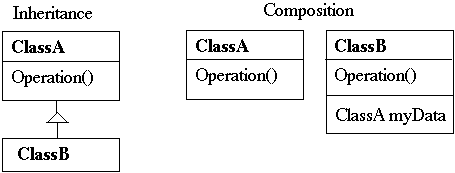
Composition
- Allows behavior changes at run time
- Helps keep classes encapsulated and focused on one task
- Reduce implementation dependencies
Parameterized Types
Generics in Ada or Eiffel
Templates in C++
Allows you to make a type as a parameter to a method or class
template <class TypeX>
TypeX min( TypeX a, Type b )
{
return a < b ? a : b;
}
Parameterized types give a third way to compose behavior in
an object-oriented system
Designing for Change
Some common design problems with design patterns that
address the problem
- Creating an object by specifying a class explicitly
- Abstract factory, Factory Method, Prototype
- Dependance on specific operations
- Chain of Responsibility, Command
- Dependence on hardware and software platforms
- Abstract factory, Bridge
- Dependence on object representations or implementations
- Abstract factory, Bridge, Memento, Proxy
- Algorithmic dependencies
- Builder, Iterator, Strategy, Template Method, Visitor
- Tight Coupling
- Abstract factory, Bridge, Chain of Responsibility,
Command, Facade, Mediator, Observer
- Extending functionality by subclassing
- Bridge, Chain of Responsibility, Composite, Decorator,
Observer, Strategy
- Inability to alter classes conveniently
- Adapter, Decorator, Visitor
visitors since 11-Feb-98