CS 596: Client-Server Programming
Spring Semester, 1997
Doc 19, CGI and Java
To Lecture Notes Index
San Diego State University -- This page last updated Apr 3, 1997

Contents of Doc 19, CGI and Java
CGI and Java | slide # 1 |
...Problems with all CGI Programs | slide # 1 |
...Problems Using Java in CGI Programs | slide # 2 |
...Solutions to Java and CGI problems | slide # 3 |
......Strawman SimpleWrapper | slide # 4 |
...Problems with SimpleWrapper | slide # 7 |
......Solution 1 - Other Libraries | slide # 7 |
......Solution 2 - One Wrapper fits all | slide # 8 |
......Solution 3 System.properties | slide # 10 |
...The Final Wrapper | slide # 11 |
...A C Equivalent of Wrapper | slide # 12 |
CGI and Java
Problems with all CGI Programs
1. Since CGI programs are run by the web server, all files,
programs and directories involved in the CGI program must
be accessable by the web server!!!!!!!!!!!!!!!!!!!!!!!
- All directories must be readable and executable by
everyone (chmod o+rx) or (chmod a+rx)
- All executables must be readable and executable by
everyone (chmod o+rx) or (chmod a+rx)
- All files must be readable by everyone (chmod o+r)
2. Data automatically available when a CGI program is run is
not automatically available when you run the program without
the broswer
- Environment variables, other input
3. When the CGI program is run by the Web server there is at
least one program between you and the CGI program
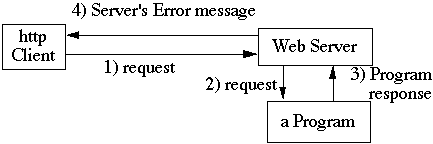
Problems Using Java in CGI Programs
1. Java can not read environment variables
- CGI requires access to environment variables
2. Java programs are started with a two word command:
- java className
- All methods of calling CGI programs (in Unix) only allow a
one word command to start the program
3. Java uses the CLASSPATH environment variable to find
classes
- CGI programs are run by the web server, not you
- Your environment is not used when your CGI program is
run
Solutions to Java and CGI problems
Use a wrapper program
Use the following java program as an example:
class HiMom
{
public static void main( String args[] ) throws Exception
{
System.out.print("Content-type: text/plain\n\n");
System.out.println("Hi Mom");
}
}
Assume java program is in file HiMom.java in directory:
- /net/www/www-eli/cgi-bin/cgiExamples
where /cgi-bin is the cgi bin directory for the web server:
- www.eli.sdsu.edu
Strawman SimpleWrapper
Put the following shell program in the file simpleWrapper in
the directory:
- /net/www/www-eli/cgi-bin/cgiExamples
The Shell program:
- #!/bin/sh
- JAVA="/opt/java/bin"
- $JAVA HiMom
Make HiMom.class and simpleWrapper world accessable:
- chmod a+rx simpleWrapper
- chmod a+r HiMom.class
Testing SimpleWrapper
Command line test:
- /net/www/www-eli -> simpleWrapper
- Content-type: text/plain
- Hi Mom
- /net/www/www-eli ->
Browserless http Test:
- /net/www/www-eli -> telnet www.eli.sdsu.edu 80
- Trying 130.191.226.80...
- Connected to www.eli.sdsu.edu.
- Escape character is '^]'.
- GET /cgi-bin/cgiExamples/simpleWrapper HTTP/1.0
- HTTP/1.0 200 OK
- Server: Netscape-Commerce/1.12
- Date: Sunday, 30-Mar-97 17:38:36 GMT
- Content-type: text/plain
- Hi Mom
- Connection closed by foreign host.
- /net/www/www-eli ->
Testing SimpleWrapper
Browser Test Access the URL:
- http://www.eli.sdsu.edu/cgi-bin/cgiExamples/simpleWrapper
Result:
- Hi Mom
Problems with SimpleWrapper
1. No environment varaibles
2. Can't use SDSU java library or other libraries not part of standard
Java distribution
3. Each CGI program requires it's own modified SimpleWrapper
program
Solution 1 - Other Libraries
The Shell program:
#!/bin/sh
- JAVA="/opt/java/bin"
- CLASSPATH=".:/opt/java/classes:/usr/local/lib/java"
- $JAVA -classpath $CLASSPATH HiMom
Solution 2 - One Wrapper fits all
Use basename and dirname
betterWrapper:
#!/bin/sh
- JAVA="/opt/java/bin"
- BASENAME="/usr/bin/basename"
DIRNAME="/usr/bin/dirname"
- CGISUFFIX=".cgi"
- CLASSPATH=".:/opt/java/classes:/usr/local/lib/java"
- CLASS=`$BASENAME $0 $CGISUFFIX`
- DIR=`$DIRNAME $0`
- cd $DIR
- $JAVA -classpath $CLASSPATH $CLASS
Using betterWrapper
Put betterWrapper into file:
- /net/www/www-eli/bin/betterWrapper
Make it world accessable and executable
Goto cgi bin directory( in my case)
- /net/www/www-eli/cgi-bin/cgiExamples
Link CGI program to betterWrapper
- ln -s ../../bin/betterWrapper HiMom
Recall that HiMom.class in is this directory
Now access the URL:
- http://www.eli.sdsu.edu/cgi-bin/cgiExamples/HiMom
Solution 3 System.properties
In Java command line arguments of the form:
- -Dname=value
Are added to the System property object
Properties is a subclass of Hashtable
Given the class Test below, the command line:
- java Test -Dname=Roger -Dclass=cs596
will produce the output:
- Roger
- cs595
class Test
{
public static void main( String args[] ) throws Exception
{
String theName = System.getProperty( "name");
Properties systemStuff = System.getProperties();
System.out.print( theName );
System.out.println( systemStuff.get( "class" ) );
}
}
The Final Wrapper
#!/bin/sh
# Java-Wrapper Version 1.1
# Copyright (C) 1996 by Marc Balmer, CH-4055 Basel, marc@msys.ch.
# This wrapper executes java binaries as CGI programs
# Modified by Roger Whitney, added suffix, DIR, Classpath, sorted and added some env variables
BASENAME="/usr/bin/basename"
DIRNAME="/usr/bin/dirname"
JAVA="/opt/java/bin"
CLASSPATH=".:/opt/java/classes:/usr/local/lib/java"
CGISUFFIX=".cgi"
CLASS=`$BASENAME $0 $CGISUFFIX`
DIR=`$DIRNAME $0`
cd $DIR
if [ -f $CLASS.class ] ; then
$JAVA -classpath $CLASSPATH \
-DAUTH_TYPE=$AUTH_TYPE \
-DCONTENT_LENGTH=$CONTENT_LENGTH \
-DCONTENT_TYPE=$CONTENT_TYPE \
-DDOCUMENT_ROOT=$DOCUMENT_ROOT \
-DGATEWAY_INTERFACE=$GATEWAY_INTERFACE \
-DHTTP_ACCEPT="$HTTP_ACCEPT" \
-DHTTP_CONNECTION=$HTTP_CONNECTION \
-DHTTP_HOST=$HTTP_HOST \
-DHTTP_USER_AGENT="$HTTP_USER_AGENT" \
-DPATH_INFO=$PATH_INFO \
-DPATH_TRANSLATED=$PATH_TRANSLATED \
-DQUERY_STRING=$QUERY_STRING \
-DREMOTE_ADDR=$REMOTE_ADDR \
-DREMOTE_HOST=$REMOTE_HOST \
-DREMOTE_IDENT=$REMOTE_IDENT \
-DREMOTE_USER=$REMOTE_USER \
-DREQUEST_METHOD=$REQUEST_METHOD \
-DSCRIPT_NAME=$SCRIPT_NAME \
-DSERVER_NAME=$SERVER_NAME \
-DSERVER_PORT=$SERVER_PORT \
-DSERVER_PROTOCOL=$SERVER_PROTOCOL \
-DSERVER_SOFTWARE=$SERVER_SOFTWARE \
$CLASS
else
echo Content-type: text/html
echo
echo \<head\>\<title\>Java-Wrapper Error\</title\>\</head\>
echo \<body\>
echo \<h1\>Java-Wrapper error\</h1\>
echo The requested java class file \<b\>$CLASS.class\</b\>
echo was not found on this server.
echo \</body\>
fi
A C Equivalent of Wrapper
A C program written by Andrew that does a bit more than
wrapper can be found at:
http://www.eli.sdsu.edu/java-SDSU/examples/net/jcgi.c
jcgi.c is slightly more general. It will send all environment
variables that starts with "HTTP_"
This difference is will not affect assignment 5.