 |
CS 635: Advanced Object-Oriented Design & Programming |
|
---|
Spring Semester, 1998
Chain of Responsibility
To Lecture Notes Index
© 1998, All Rights Reserved, SDSU & Roger Whitney
San Diego State University -- This page last updated 23-Apr-98
Contents of Doc 25, Chain of Responsibility
CS 635 Doc 25 Chain of Responsibility
References | slide # 1 |
| |
Chain of Responsibility | slide # 2 |
...Intent | slide # 2 |
...Participants | slide # 3 |
...Motivation | slide # 4 |
...When to Use | slide # 4 |
...Implementation Issues | slide # 6 |
References
Design Patterns: Elements of Resuable Object-Oriented
Software, Gamma, Helm, Johnson, Vlissides, Addison-Wesley,
1995, pp 223-232
Chain of Responsibility
Intent
Avoid coupling the sender of a request to its receiver by giving
more than one object a chance to handle the request. Chain the
receiving objects and pass the request along the chain until an
object handles it.
Class Structure
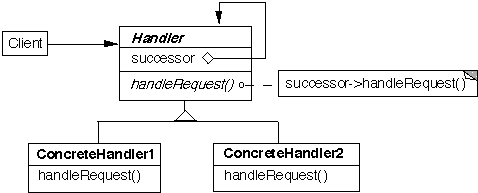
Sample Object Structure

Participants
Handler
- Defines the interface for handling the requests
- May implement the successor link
ConcreteHandler
- Handles requests it is responsible for
- Can access its successor
- Handles the request if it can do so, otherwise it forwards the
request to its successor
Consequences
- Reduced coupling
- Added flexibility in assigning responsibilities to objects
- Not guaranteed that request will be handled
Motivation
Context Help System
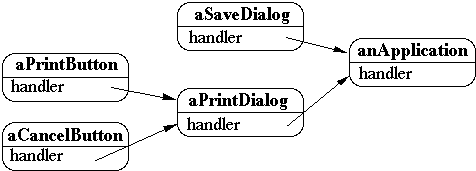
When to Use
When more than on object may handle a request, and the
handler isn't known a priori
When you want to issue a request to one of several objects
without specifying the receiver explicitly
When the set of objects that can handle a request should be
specified dynamically
How does this differ from Decorator?
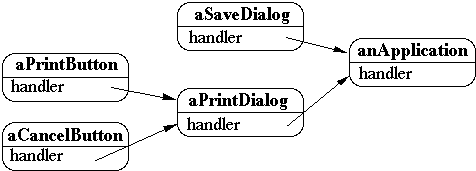

Implementation Issues
The successor chain
- Use existing links in the program
- The concrete handlers may already have pointers to their
successors, so just use them
- Give each handler a link to its successor
Representing Requests
- Each request can be a hard-coded
abstract class HardCodedHandler
{
private HardCodedHandler successor;
public HardCodedHandler( HardCodedHandler aSuccessor)
{ successor = aSuccessor; }
public void handleOpen()
{ successor.handleOpen(); }
public void handleClose()
{ successor.handleClose(); }
public void handleNew( String fileName)
{ successor.handleClose( fileName ); }
}
- A single method implements all requests
abstract class SingleHandler
{
private SingleHandler successor;
public SingleHandler( SingleHandler aSuccessor)
{
successor = aSuccessor;
}
public void handle( String request)
{
successor.handle( request );
}
}
class ConcreteOpenHandler extends SingleHandler
{
public void handle( String request)
{
switch ( request )
{
case "Open" : do the right thing;
case "Close" : more right things;
case "New" : even more right things;
default: successor.handle( request );
}
}
}
- Single handle method with Request Object for parameters
abstract class SingleHandler
{
private SingleHandler successor;
public SingleHandler( SingleHandler aSuccessor)
{successor = aSuccessor; }
public void handle( Request data)
{ successor.handle( data ); }
}
class ConcreteOpenHandler extends SingleHandler
{
public void handle( Open data)
{ // handle the open here }
}
class Request
{
private int size;
private String name;
public Request( int mySize, String myName)
{ size = mySize; name = myName; }
public int size() { return size; }
public String name() { return name;}
}
class Open extends Request
{// add Open specific stuff here}
class Close extends Request
{ // add Close specific stuff here}
© 1998, All Rights Reserved, SDSU & Roger Whitney
visitors since 23-Apr-98