 |
CS 635: Advanced Object-Oriented Design & Programming |
|
---|
Spring Semester, 1998
Facade and Mediator
To Lecture Notes Index
© 1998, All Rights Reserved, SDSU & Roger Whitney
San Diego State University -- This page last updated 23-Apr-98
Contents of Doc 26, Facade and Mediator
CS 635 Doc 26 Facade & Mediator
References | slide # 1 |
| |
Facade | slide # 2 |
...Review Wirfs-Brock CRC Design Process | slide # 2 |
......Exploratory Phase | slide # 3 |
......Analysis Phase | slide # 4 |
......Subsystems | slide # 5 |
...The Facade Pattern - Basic Idea | slide # 6 |
...Consequences of Facade Pattern | slide # 6 |
| |
Mediator | slide # 7 |
...Structure | slide # 7 |
...Participants | slide # 8 |
...Motivating Example | slide # 9 |
...When to use the Mediator Pattern | slide # 10 |
...Issues | slide # 11 |
References
Designing Object-Oriented Software,Wirfs-Brock
Design Patterns: Elements of Resuable Object-Oriented
Software, Gamma, Helm, Johnson, Vlissides, Addison
Wesley, 1995, pp 185-194
Facade
Review of Wirfs-Brock (CRC Design Process)
- Who is on the team?
- What are their tasks, responsibilities?
- Who works with whom?
- Who's related to whom?
- Finding sub teams
- Putting it all together
Exploratory Phase
- What are the goals of the system?
- What must the system accomplish?
- What objects are required to model the system and
accomplish the goals?
- What are their tasks, responsibilities?
- What does each object have to know in order to accomplish
each goal it is involved with?
- What steps toward accomplishing each goal is it
responsible for?
- With whom will each object collaborate in order to
accomplish each of its responsibilities?
- What is the nature of the objects' collaboration
Analysis Phase
- Determine which classes are related via inheritance
- Finding abstract classes
- Determine class contracts
- Divide responsibilities into subsystems
- Designing interfaces of subsystems and classes
- Construct protocols for each class
- Produce a design specification for each class and
subsystem
- Write a design specification for each contract
Subsystems
Subsystems are groups of classes, or groups of classes and
other subsystems, that collaborate among themselves to support
a set of contracts
There is no conceptual difference between the responsibilities of
a class and a subsystem of classes
The difference between a class and subsystem of classes is a
matter of scale
A subsystem should be a good abstraction
There should be as little communication between different
subsystems as possible
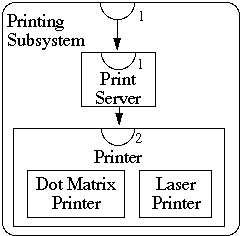
The Facade Pattern - Basic Idea
Create a class that is the interface to the subsystem
Clients interface with the Facade class to deal with the
subsystem
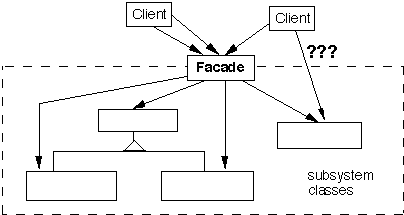
Consequences of Facade Pattern
It hides the implementation of the subsystem from clients
It promotes weak coupling between the subsystems and its
clients
It does not prevent clients from using subsystems class, should
it?
Mediator
A mediator is responsible for controlling and coordinating the
interactions of a group of objects (not data structures)
Structure
Classes
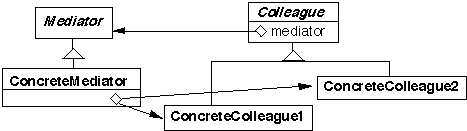
Objects
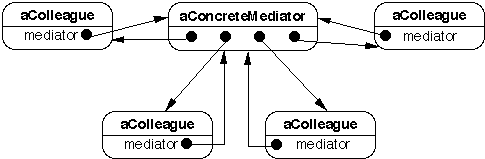
Participants
Mediator
- Defines an interface for communicating with Colleague objects
ConcreteMediator
- Implements cooperative behavior by coordinating Colleague
objects
- Knows and maintains its colleagues
Colleague classes
- Each Colleague class knows its Mediator object
- Each colleague communicates with its mediator whenever it
would have otherwise communicated with another colleague
Motivating Example
Dialog Boxes
Objects
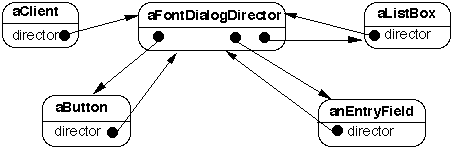
Interaction
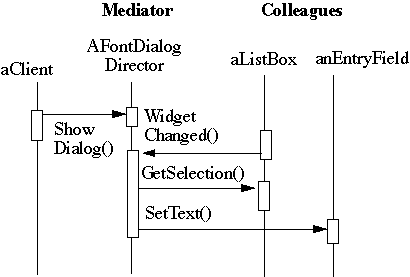
How does this differ from a God Class?
When to use the Mediator Pattern
When a set of objects communicate in a well-defined but
complex ways
When reusing an object is difficult because it refers to and
communicates with many other objects
When a behavior that's distributed between several classes
should be customizable without a lot of subclassing
Issues
How do Colleagues and Mediators Communicate?
1) Explicit methods in Mediator
class DialogDirector
{
private Button ok;
private Button cancel;
private ListBox courses;
public void ListBoxItemSelected() { blah}
public void ListBoxScrolled() { blah }
etc.
}
2) Generic change method
class DialogDirector {
private Button ok;
private Button cancel;
private ListBox courses;
public void widgetChanged( Object changedWidget) {
if ( changedWidget == ok ) blah
else if ( changedWidget == cancel ) more blah
else if ( changedWidget == courses ) even more blah
}
}
3) Generic change method overloaded
class DialogDirector
{
private Button ok;
private Button cancel;
private ListBox courses;
public void widgetChanged( Button changedWidget)
{
if ( changedWidget == ok )
blah
else if ( changedWidget == cancel )
more blah
}
public void widgetChanged( ListBox changedWidget)
{
now find out how it changed and
respond properly
}
}
Differences from Facade
Facade does not add any functionality, Mediator does
Subsystem components are not aware of Facade
Mediator's colleagues are aware of Mediator and interact with it
© 1998, All Rights Reserved, SDSU & Roger Whitney
visitors since 23-Apr-98