CS535 Object-Oriented Programming & Design
Fall Semester, 1996
Doc 11, Inheritance & Polymorphism
[To Lecture Notes Index]
San Diego State University -- This page last updated Oct 7, 1996

Contents of Doc 11, Inheritance & Polymorphism
- References
- Inheritance and Polymorphism
- Simplistic Example
- Finding Classes
- Relationships Between Classes
- Banking Classes
- Avoid Case (and if) Statements
- How to Increase the use of Polymorphism4
- Grow Well Shaped & Balanced Trees
- Metric Rules of Thumb5
R. J. Abbott, "Programming Design by Informal English Descriptions",
Communications of the ACM, Vol. 26, No 11, November 1983, pp. 882 -
894
Grady Booch, Object-Oriented Analysis and Design, 1994
Mark Lorenz, Object-Oriented Software Development: A Practical Guide,
1993, Appendix I Measures and Metrics
Johnson, Ralph E. and Foote, Brain, "Designing Reusable Classes," Journal of
Object-Oriented Programming, pages 22-35, June/July 1988.
Shlaer, S. and Mellor, S.J., Object-Oriented Systems Analysis: Modeling the
World In Data, Yourdon Press: Prentice-Hall, Englewood Cliffs, New Jersey,
1988.
Last Federal Virtual Bank wants its entire banking software redone in Java, so
it can shut down its branch offices and perform all its business via the WWW.
Customer records include the customers name, address, personal information,
passwords, etc. and accounts.
The bank offers various types of accounts: checking, savings, CD, Junior
savings accounts.
Bank officers will introduce new types of accounts from time to time.
Checking account charge 12 cents/check unless the average account balance for
the month is greater than $1,000. The first three ATM transactions per month
are free. Additional ATM transactions cost 50 cents.
All transactions over $1,000 dollars must be reported to the federal
government.
Junior savings accounts are for children under the age of 13. Children can
deposit any amount into the account. Deposits of over $100 are reported to
their parents. Children can withdrawal up to $10 from the account. Individual
withdrawals over $10 must be verified by a parent. Total withdrawals over $100
per month are reported to the parents.
Transactions include time & date, amount, location, type of transaction,
account number and teller.
Abbot[1]
"In an English description of the problem, the nouns are candidates for
objects; the verbs are candidates for operations."
Look at these phrases. Some will be obvious classes, some will be obvious
nonsense, and some will fall between obvious and nonsense. Skip the nonsense,
keep the rest. The goal is a list of candidate objects. Some items in the
list will be eliminated, others will be added later. Finding good objects is a
skill, like finding a good functional decomposition.
Booch[2]
"The tangible things in problem domain, the roles they play, and the events
that may occur form the candidate classes and object of our design."
- Disks
- Printers Airplanes
- Model conceptual entities that form a cohesive abstraction
- Window
- File Bank Account
- If more than one word applies to a concept select the one that is most
meaningful
- Model categories of classes
- Categories may become abstract classes
-
- Keep them as individual classes at this point
- Model known interfaces to outside world
- User interfaces
-
- Interfaces to other programs
-
- Model the values of attributes, not the attributes themselves
- Height of a rectangle
Height is an attribute of rectangle
Value of height is a number
Rectangle can record its height
Finding Classes Other Views
Coad and Yourdon
Structure
-
- "Kind of" and "part of" relationships
Other systems
-
- External systems with which the application interacts
Devices
-
- Devices with which the application interacts
Events remembered
-
- Historical event that must be recorded
Roles played
-
- Different roles users play in interacting with application
Locations
-
- Physical locations, offices and sites important to the application
Ross
People
-
- Humans who carry out some function
- Farmers
Places
-
- Areas set aside for people or things
-
- Fields on farm
Things
- Physical objects or groups of objects
-
- Plants
- leaves roots
Organizations
- Formally organized collections of people, resources, facilities
-
- Union
- farm crew
Concepts
- Principles or ideas not tangible
Events
- Things that happen
Shaler & Mellor[3]
Tangible things
-
- Cars
- telemetry data
Roles
-
- Mother
- teacher
Events
-
- Landing
- interrupt
Interactions
-
- Loan
- meeting
Is-kind-of or is-a
- Implies inheritance
-
- Place common responsibilities in superclass
is-analogous-to
- If class X is-analogous-to class Y then look for superclass
is-part-of or has-a
-
- If class A is-part-of class B then there is no inheritance
-
- Some negotiation between A and B for responsibilities may be needed
-
- Example:
Assume A contains a list that B uses
Who sorts the list? A or B?
Customer
Transaction
Currency
Account
abstract class account {
public static final boolean VALID = true;
protected Customer accountOwner;
protected Currency balance;
protected Stack accountHistory;
// Other fields left out
public void processTransaction( Transaction accountActivity ) {
if ( validateTransaction( accountActivity ) == VALID )
commitTransaction( accountActivity );
else
// report the problem. Code not shown
}
protected abstract boolean validateTransaction( Transaction
accountActivity
);
protected abstract void commitTransaction( Transaction
accountActivity
);
// other methods left out
}
Account Class Inheritance
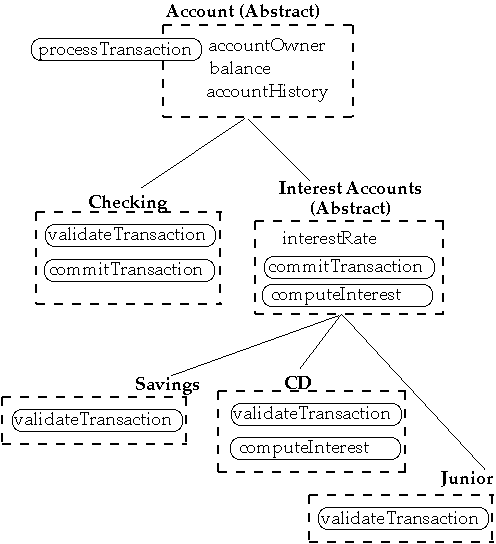
Polymorphism
Account newCustomer;
newCustomer = magicFunctionToCreateNewAccount()
newCustomer.processTransaction( amount );
Which
processTransaction is called?
Adding new types of accounts to program requires:
- Adding new subclasses
-
- Changing code that creates objects
Modular Design Rules
switch ( newCustomer.accountType ) {
case SAVINGS:
// Code to process Savings account
case CD:
// Code to process CD account
case CHECKING:
// Code to process Checking account
// etc.
}
Use Polymorphism
newCustomer.processTransaction( amount );
Supports:
Composability Continuity
Protocol
- Name and arguments of public operations of an object
Plug Compatible Objects
- Objects with the same protocol
-
-
Standard Protocol
Using the same name for similar processes
Rules for Finding Standard Protocols
(To Encourage Polymorphism)
- Smalltalk methods average about 3 - 5 lines of code
Rules for Finding Standard Protocols
(To Encourage Polymorphism)
- Reduce the number of arguments
- Methods with small number of arguments are more likely to be usable in
different classes
-
-
- Reduce number of arguments by:
-
- Breaking a message into several smaller messages
-
- Creating a new class that represents a group of arguments
Rules for Finding Standard Protocols
(To Encourage Polymorphism)
- Checking class of an object
if (anObject class = integer) then
anObject.Foo;
else
anObject.Fee;
- Implement Foo in all relevant classes to perform the equivalent operation
anObject.Foo
- Case analysis of values of variables
- Can be more difficult, but no less important
Rules for Finding Standard Protocols
(To Encourage Polymorphism)
- If an operation X is implemented by performing a similar operation on the
component of the receiver, then that operation should also be named X.
-
-
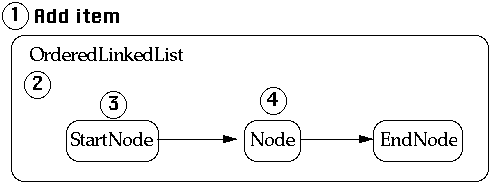
void OrderedLinkedList::add(int value)
{
cell *newCell;
newCell = new cell;
newCell->value = value;
listHead->add(*newCell);
};
Modular Design Rules
Avoid Short Fat Trees
Avoid Tall Skinny Trees
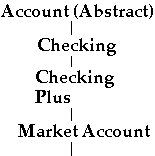
- The average method size should be less than 8 lines of code (LOC) for
Smalltalk and 24 LOC for C++
- Bigger averages indicate object-oriented design problems
- The average number of methods per class should be less than 20
- Bigger averages indicate too much responsibility in too few
classes
- The average number of fields per class should be less than 6.
- Bigger averages indicate that one class is doing more than it
should
- The class hierarchy nesting level should be less than 6
- Start counting at the level of any framework classes you use or the root
class if you don't
- The average number of comment lines per method should be greater than 1