CS 596 OODP
Xforms Introduction
[To Lecture Notes Index]
San Diego State University -- This page last updated Oct. 12, 1995

Contents of Xforms Introduction Lecture
- References
- Misc.
- Form Designer -GUI builder
- First Example
- The Basic Steps
- Initialize Form
- Defining Forms
- Showing Forms
- Main Loop
- Things that can go on a Form
- Boxes
- Text
- Buttons
- Sliders
- Input fields
- Grouping Objects
- Hiding and Showing Objects
- Deactivating and reactivating Objects
- Changing Attributes
- Color
- Bounding Boxes
- Label (Text) Attributes
- Some Goodies
- Interaction - Callbacks
- Callback with Objects
Forms Library: A Graphical User Interface Toolkit for X by T. C. Zhao and Mark
Overmars., 1995
More information
http://bragg.phys.uwm.edu/xforms
Examples
/opt/xforms/DEMOS
Manual
ftp://bragg.phys.uwm.edu/xforms/DOC
Cal Copy
Compiling
g++ -I/opt/xforms/FORMS -I/opt/X11R5/lib
fileName.c
-L/opt/xforms/FORMS -lforms
-L/opt/X11R5/lib -lX11 -lnsl -lsocket -lm
Options
-I (uppercase i) location to find include files
-L (uppercase L) location to find libraries
-l (lowercase L) names of the libraries program needs to be linked to
Two Line Compilation
g++ -O -I/opt/xforms/FORMS -I/opt/X11R5/lib
-c fileName.c
g++ -O -o fileName fileName.o
-L/opt/xforms/FORMS -lforms
-L/opt/X11R5/lib -lX11 -lnsl -lsocket -lm
Magic Makefile
CFLAGS = -O
LIB = -L/opt/xforms/FORMS -lforms \
-L/opt/X11R5/lib -lX11 \
-lnsl -lsocket -lm
INCL = -I/opt/xforms/FORMS -I/opt/X11R5/lib
CC = g++
.c.o:
$(CC) $(CFLAGS) $(INCL) -c $*.c
.o:
$(CC) $(CFLAGS) -o $* $*.o $(LIB)
.c:
$(CC) $(CFLAGS) $(INCL) -c $*.c
$(CC) $(CFLAGS) -o $* $*.o $(LIB)
clean:
rm *.o
Sample Use
Put program in file named Bank.c
Then following command compiles program
make Bank
Warning
Xforms library works with C and C++
Xforms library uses object/class concepts but does not use C++ classes
Programs compiled with C++ must have the following header.
- #include <string.h>
- #include "forms.h"
#include <string.h> must come first
string.h for CC is sick, Do not use CC until string.h is fixed
Use g++ for now
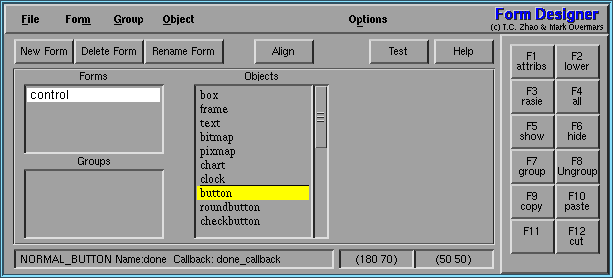
#include <string.h>
#include "forms.h"
int main( int argc, char *argv[] )
{
FL_FORM *simpleform;
fl_initialize( argv[0], "FormDemo", 0, 0, &argc, argv );
simpleform = fl_bgn_form( FL_UP_BOX, 230, 160 );
fl_add_button( FL_NORMAL_BUTTON,
40, 50, 150, 60,
"Push Me" );
fl_end_form( );
fl_show_form( simpleform, FL_PLACE_MOUSE,
FL_NOBORDER, NULL );
fl_do_forms( );
fl_hide_form( simpleform );
return 1;
}
Initialize the Forms Library
Defining Forms
Showing Forms
Main Loop
fl_initialize( argv[0], "FormDemo", 0, 0, &argc, argv );
FL_FORM* fl_bgn_form( FormType, width , height );
// Define form here
fl_end_form( );
FormType type of background of form
- FL_UP_BOX
- Comes out of the screen
- FL_DOWN_BOX
- Goes down into the screen
- FL_FLAT_BOX
- Flat box with no border
- FL_BORDER_BOX
- Flat box with border
- FL_FRAME_BOX
- Flat box with engraved border
- FL_SHADOW_BOX
- Flat box with a shadow
- FL_ROUNDED_BOX
- Rounded box
- FL_RFLAT_BOX
- Rounded box without border
- FL_RSHADOW_BOX
- Rounded box with shadow
- FL_OVAL_BOX
- Box shaped like an ellipse
- FL_NO_BOX
- No box at all
width Width of form in pixels
height Height of form in pixels
simpleform = fl_bgn_form( FL_UP_BOX, 230, 160 );
fl_add_button( FL_NORMAL_BUTTON,
40, 50, 150, 60,
"Push Me" );
fl_end_form( );
long fl_show_form( FormPointer, InitialLocation,
BorderType, const char* Title );
Show form on the screen
InitialLocation
FL_PLACE_SIZE User can control location, not size
FL_PLACE_MOUSE Place below mouse
FL_PLACE_CENTER Centered in the screen
FL_PLACE_FULLSCREEN Cover the entire screen
FL_PLACE_FREE Position and size are free
See page 29-30 of manual for more options
Option can be combined
- FL_PLACE_MOUSE | FL_PLACE_FREE
BorderType
FL_FULLBORDER Full border with title
FL_TRANSIENT Less decoration
FL_NOBORDER no border at all
fl_show_form( simpleform, FL_PLACE_MOUSE,
FL_NOBORDER, NULL );
fl_do_forms( );
returns when user changes state of the form
First Example Again
#include <string.h>
#include "forms.h"
int main( int argc, char *argv[] )
{
FL_FORM *simpleform;
fl_initialize( argv[0], "FormDemo", 0, 0, &argc, argv );
simpleform = fl_bgn_form( FL_UP_BOX, 230, 160 );
fl_add_button( FL_NORMAL_BUTTON,
40, 50, 150, 60,
"Push Me" );
fl_end_form( );
fl_show_form( simpleform, FL_PLACE_MOUSE,
FL_NOBORDER, NULL );
fl_do_forms( );
fl_hide_form( simpleform );
return 1;
}
Multiple Forms
#include <iostream.h>
#include <string.h>
#include "forms.h"
int main( int argc, char *argv[] )
{
FL_FORM* niceForm;
FL_FORM* meanForm;
fl_initialize( argv[0], "FormDemo", 0, 0, &argc, argv );
niceForm = fl_bgn_form( FL_UP_BOX, 150, 100 );
fl_add_button( FL_NORMAL_BUTTON,
40, 50, 100, 60, "Please Push Me" );
fl_end_form( );
meanForm = fl_bgn_form( FL_UP_BOX, 150, 100 );
fl_add_button( FL_NORMAL_BUTTON,
40, 50, 100, 60, "Push Me or Else!" );
fl_end_form( );
fl_show_form( niceForm, FL_PLACE_MOUSE,
FL_TRANSIENT, "Nice" );
fl_show_form( meanForm, FL_PLACE_CENTER,
FL_FULLBORDER, "Watch Out!" );
cout << "Get ready to Start" << endl;
fl_do_forms( );
cout << "User selected something" << endl;
fl_hide_form( niceForm);
fl_do_forms( );
cout << "The End " << endl;
return 1;
}
Keeping Track of Buttons
int main( int argc, char *argv[] )
{
FL_FORM *form;
FL_OBJECT *yes, *no, *button;
fl_initialize( argv[0], "FormDemo", 0, 0 , &argc, argv );
form = fl_bgn_form( FL_UP_BOX, 320, 120 );
fl_add_box( FL_NO_BOX, 160, 80, 0, 0,
"Do you want to Quit?" );
yes = fl_add_button( FL_NORMAL_BUTTON,
40, 20, 80, 30, "Yes" );
no = fl_add_button( FL_NORMAL_BUTTON,
200, 20, 80, 30, "No" );
fl_end_form( );
fl_show_form( form, FL_PLACE_MOUSE,
FL_NOBORDER, NULL );
do
button= fl_do_forms( );
while ( button != yes );
fl_hide_form( form );
return 0;
}
FL_OBJECT* fl_add_box(boxType, XCoordinate, YCoordinate,
width, height, label );
- FL_UP_BOX
- Comes out of the screen
- FL_DOWN_BOX
- Goes down into the screen
- FL_FLAT_BOX
- Flat box with no border
- FL_BORDER_BOX
- Flat box with border
- FL_FRAME_BOX
- Flat box with engraved border
- FL_SHADOW_BOX
- Flat box with a shadow
- FL_ROUNDED_BOX
- Rounded box
- FL_RFLAT_BOX
- Rounded box without border
- FL_RSHADOW_BOX
- Rounded box with shadow
- FL_OVAL_BOX
- Box shaped like an ellipse
- FL_NO_BOX
- No box at all
Box Example
#include <string.h>
#include "forms.h"
int main( int argc, char *argv[] )
{
FL_FORM *boxExample;
FL_OBJECT plainBox;
FL_OBJECT fancyBox;
fl_initialize( argv[0], "FormDemo", 0, 0, &argc, argv );
boxExample = fl_bgn_form( FL_UP_BOX, 150, 150 );
myBox = fl_add_box( FL_BORDER_BOX,
10, 10, 100, 50, "Plain Jane" );
fancyBox = fl_add_box( FL_FRAME_BOX,
10, 70, 100, 50, "\010Look Out" );
// set box color
fl_set_object_color( fancyBox, FL_RED, 0 );
// set label color
fl_set_object_lcol( fancyBox, FL_GREEN );
// set label font size
fl_set_object_lsize( fancyBox, FL_LARGE_SIZE );
fl_end_form( );
fl_show_form( boxExample, FL_PLACE_MOUSE,
FL_FULLBORDER, "Boxes" );
fl_do_forms( );
fl_hide_form( boxExample);
return 1;
}
FL_OBJECT* fl_add_text( textType, XCoordinate, YCoordinate,
width, height, label );
textType = FL_NORMAL_TEXT
Fancy Stuff?
\010 in front of text underlines all text
- "\010Hi Mom"
- Hi Mom
\010 in side of text, underlines next character
"Hi \010Mom" Hi Mom
\n in text gives newline
FL_OBJECT* fl_add_button( buttonType, XCoordinate,
YCoordinate, width, height, label );
FL_OBJECT* fl_add_lightbutton( buttonType, XCoordinate,
YCoordinate, width, height, label );
FL_OBJECT* fl_add_roundbutton( buttonType, XCoordinate,
YCoordinate, width, height, label );
FL_OBJECT* fl_add_checkbutton( buttonType, XCoordinate,
YCoordinate, width, height, label );
FL_OBJECT* fl_add_bitmapbutton( buttonType, XCoordinate,
YCoordinate, width, height, label );
FL_OBJECT* fl_add_pixmapbutton( buttonType, XCoordinate,
YCoordinate, width, height, label );
Some Button Types
FL_NORMAL_BUTTON Returns value when released
FL_PUSH_BUTTON Stays pushed until pushed again
FL_TOUCH_BUTTON Returns value as long as it is pushed
FL_RADIO_BUTTON Switches off other radio buttons
FL_INOUT_BUTTON Returns value when pushed and released
FL_RETURN_BUTTON Like normal button but reacts on return
PushButton.c - An Example of Buttons
#include <string.h>
#include "forms.h"
FL_FORM *form;
FL_OBJECT *button[8], *box[8], *exitButton;
void makeform( void )
{
int i;
form = fl_bgn_form( FL_UP_BOX, 400, 400 );
for ( i=0; i<8; i++ )
{
button[i] = fl_add_button( FL_PUSH_BUTTON,
40, 60+40*i, 80, 30, "" );
fl_set_object_color( button[i], FL_BLACK+i+1,
FL_BLACK+i+1 );
box[i] = fl_add_box( FL_DOWN_BOX, 150+30*i,
40, 25, 320, "" );
fl_set_object_color( box[i], FL_BLACK+i+1,
FL_BLACK+i+1 );
fl_hide_object( box[i] );
}
exitButton = fl_add_button( FL_NORMAL_BUTTON,
40, 20, 80, 30, "Exit" );
fl_end_form( );
}
int
main( int argc, char *argv[] )
{
FL_OBJECT *obj;
int i;
fl_initialize( argv[0], "FormDemo", 0, 0 , &argc, argv );
makeform( );
fl_show_form( form, FL_PLACE_CENTER,
FL_NOBORDER, NULL );
do
{
obj = fl_do_forms( );
for ( i=0; i<8; i++ )
if ( obj == button[i] )
if ( box[i]->visible )
fl_hide_object( box[i] );
else
fl_show_object( box[i] );
} while ( obj != exitButton );
fl_hide_form( form );
return 0;
}
Demo 04 In case you ask
The
following code, demo04.c, creates the above window.
Note this is created with a GUI builder
#include "forms.h"
FL_FORM *buttons;
FL_OBJECT *readyobj;
#include "nomail.xbm"
#include "newmail.xbm"
#include "crab.xpm"
#include "crab45.xpm"
static void bitmapbutton( FL_OBJECT *ob, long q )
{
int set = fl_get_button( ob );
fl_set_bitmapbutton_data( ob, newmail_width,
newmail_height,
set ? nomail_bits:newmail_bits );
}
static void pixmapbutton( FL_OBJECT *ob, long q )
{
int set = fl_get_button( ob );
fl_set_pixmapbutton_data( ob, set ? crab45:crab );
}
void create_form_buttons( void )
{
FL_OBJECT *obj;
if ( buttons )
return;
buttons = fl_bgn_form( FL_NO_BOX, 320, 380 );
obj = fl_add_box( FL_UP_BOX, 0, 0, 320, 380, "" );
readyobj = obj = fl_add_button( FL_NORMAL_BUTTON,
190, 15, 110, 30, "READY" );
obj = fl_add_button( FL_NORMAL_BUTTON,
125, 315, 150, 35, "Button" );
fl_set_object_boxtype( obj, FL_SHADOW_BOX );
fl_set_object_color( obj, FL_GREEN, FL_RED );
obj = fl_add_bitmapbutton( FL_PUSH_BUTTON,
180, 100, 80, 70, "" );
fl_set_object_boxtype( obj, FL_NO_BOX );
fl_set_object_callback( obj, bitmapbutton, 0 );
fl_set_bitmapbutton_data( obj, newmail_width,
newmail_height, newmail_bits );
obj = fl_add_pixmapbutton( FL_PUSH_BUTTON,
200, 60, 45, 40, "" );
fl_set_object_callback( obj, pixmapbutton, 0 );
fl_set_pixmapbutton_data( obj, crab );
fl_bgn_group( );
obj = fl_add_roundbutton( FL_RADIO_BUTTON,
25, 300, 40, 50, "" );
fl_set_object_boxtype( obj, FL_BORDER_BOX );
fl_set_object_color( obj, FL_MCOL, FL_RED );
obj = fl_add_roundbutton( FL_RADIO_BUTTON,
25, 255, 40, 50, "" );
fl_set_object_boxtype( obj, FL_BORDER_BOX );
fl_set_object_color( obj, FL_MCOL, FL_GREEN );
obj = fl_add_roundbutton( FL_RADIO_BUTTON,
25, 205, 40, 50, "" );
fl_set_object_boxtype( obj, FL_BORDER_BOX );
fl_set_object_color( obj, FL_MCOL, FL_BLUE );
fl_end_group( );
fl_bgn_group( );
obj = fl_add_frame( FL_ENGRAVED_FRAME,
115, 205, 75, 95, "" );
obj = fl_add_box( FL_FLAT_BOX,
125, 295, 40, 10, "CheckB" );
obj = fl_add_checkbutton( FL_RADIO_BUTTON,
120, 265, 55, 30, "Red" );
fl_set_object_color( obj, FL_MCOL, FL_RED );
obj = fl_add_checkbutton( FL_RADIO_BUTTON,
120, 235, 55, 30, "Green" );
fl_set_object_color( obj, FL_MCOL, FL_GREEN );
obj = fl_add_checkbutton( FL_RADIO_BUTTON,
120, 205, 55, 30, "Blue" );
fl_set_object_color( obj, FL_MCOL, FL_BLUE );
fl_end_group( );
fl_bgn_group( );
obj = fl_add_lightbutton( FL_RADIO_BUTTON,
20, 130, 125, 35, " Red" );
fl_set_object_color( obj, FL_COL1, FL_RED );
obj = fl_add_lightbutton( FL_RADIO_BUTTON,
20, 95, 125, 35, " Green" );
fl_set_object_color( obj, FL_COL1, FL_GREEN );
obj = fl_add_lightbutton( FL_RADIO_BUTTON,
20, 60, 125, 35, " Blue" );
fl_set_object_color( obj, FL_COL1, FL_BLUE );
fl_end_group( );
fl_bgn_group( );
obj = fl_add_roundbutton( FL_RADIO_BUTTON,
230, 265, 55, 30, "Red" );
fl_set_object_color( obj, FL_MCOL, FL_RED );
obj = fl_add_frame( FL_SHADOW_FRAME,
225, 205, 70, 95, "" );
obj = fl_add_roundbutton( FL_RADIO_BUTTON,
230, 205, 55, 30, "Blue" );
fl_set_object_color( obj, FL_MCOL, FL_BLUE );
obj = fl_add_roundbutton( FL_RADIO_BUTTON,
230, 235, 55, 30, "Green" );
fl_set_object_color( obj, FL_MCOL, FL_GREEN );
obj = fl_add_roundbutton( FL_RADIO_BUTTON,
230, 265, 55, 30, "Red" );
fl_set_object_color( obj, FL_MCOL, FL_RED );
fl_end_group( );
fl_end_form( );
}
int
main( int argc, char *argv[] )
{
fl_initialize( argv[0], "FormDemo", 0, 0, &argc, argv );
create_form_buttons( );
fl_show_form( buttons, FL_PLACE_CENTER,
FL_NOBORDER, 0 );
while ( fl_do_forms( ) != readyobj );
;
return 0;
}
FL_OBJECT* fl_add_slider( sliderType, XCoordinate,
YCoordinate, width, height, label );
void fl_set_slider_value( FL_OBJECT* aSlider, double value );
void fl_set_slider_bounds( FL_OBJECT* aSlider,
double minValue, double maxValue );
double fl_get_slider_value( FL_OBJECT* aSlider );
sliderType
FL_VERT_SLIDER
FL_HOR_SLIDER
#include <iostream.h>
#include <string.h>
#include "forms.h"
int main( int argc, char *argv[] )
{
FL_FORM* form;
FL_OBJECT* slider;
fl_initialize( argv[0], "FormDemo", 0, 0, &argc, argv );
form = fl_bgn_form( FL_UP_BOX, 150, 190 );
slider = fl_add_slider( FL_VERT_SLIDER, 30, 30, 60,
120, "" );
fl_set_slider_bounds( slider, -1, 1 );
fl_set_slider_value( slider, 0 );
fl_end_form( );
fl_show_form( form, FL_PLACE_CENTER,
FL_FULLBORDER, "Slide" );
cout << "Get ready to Start" << endl;
fl_do_forms( );
cout << "The value is" << fl_get_slider_value( slider ) << endl;
fl_hide_form( form);
return 1;
}
Input fields supports full emacs style editing
FL_OBJECT* fl_add_input( inputType, XCoordinate,
YCoordinate, width, height, label );
void fl_set_input( FL_OBJECT* input, char* default text );
void fl_set_input_color( FL_OBJECT* input, textColor, cursorColor );
[const] char* fl_get_input( FL_OBJECT* input );
void fl_set_object_focus( FL_FORM* aForm, FL_OBJECT* input );
inputType
FL_NORMAL_INPUT Any text can be typed
FL_FLOAT_INPUT Only float values can be typed
FL_INT_INPUT Only int values can be typed
FL_MULTILINE_INPUT Allows for multiple lines
FL_SECRET_INPUT Does not show text typed
FL_HIDDEN_INPUT Input field is invisible
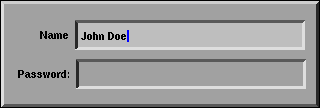
FL_OBJECT* fl_bgn_group(void)
void fl_end_group(void)
#include <string.h>
#include "forms.h"
int main( int argc, char *argv[] )
FL_FORM *buttons;
buttons = fl_bgn_form( FL_NO_BOX, 320, 380 );
fl_bgn_group( );
fl_add_roundbutton( FL_RADIO_BUTTON,
25, 300, 40, 50, "First" );
fl_add_roundbutton( FL_RADIO_BUTTON,
25, 255, 40, 50, "Second" );
fl_add_roundbutton( FL_RADIO_BUTTON,
25, 205, 40, 50, "Third" );
fl_end_group( );
fl_end_form( );
fl_show_form( buttons , FL_PLACE_MOUSE,
FL_FULLBORDER, "buttons " );
fl_do_forms( );
fl_hide_form( buttons );
return 1;
}
void fl_hide_object( FL_OBJECT* aThing )
void fl_show_object( FL_OBJECT* aThing )
int main( int argc, char *argv[] )
{
FL_FORM *form;
FL_OBJECT *yes, *no, *button;
fl_initialize( argv[0], "FormDemo", 0, 0 , &argc, argv );
form = fl_bgn_form( FL_UP_BOX, 320, 120 );
fl_add_box( FL_NO_BOX, 160, 80, 0, 0,
"Do you want to Quit?" );
yes = fl_add_button( FL_NORMAL_BUTTON,
40, 20, 80, 30, "Yes" );
no = fl_add_button( FL_NORMAL_BUTTON,
200, 20, 80, 30, "No" );
fl_end_form( );
fl_hide_object( yes )
fl_show_form( form, FL_PLACE_MOUSE,
FL_NOBORDER, NULL );
fl_do_forms( );
fl_show_object( yes );
do
button= fl_do_forms( );
while ( button != yes );
fl_hide_form( form );
return 0;
}
void fl_deactivate_object( FL_OBJECT* aThing )
void fl_activate_object( FL_OBJECT* aThing )
int main( int argc, char *argv[] )
{
FL_FORM *form;
FL_OBJECT *yes, *no, *button;
fl_initialize( argv[0], "FormDemo", 0, 0 , &argc, argv );
form = fl_bgn_form( FL_UP_BOX, 320, 120 );
fl_add_box( FL_NO_BOX, 160, 80, 0, 0,
"Do you want to Quit?" );
yes = fl_add_button( FL_NORMAL_BUTTON,
40, 20, 80, 30, "Yes" );
no = fl_add_button( FL_NORMAL_BUTTON,
200, 20, 80, 30, "No" );
fl_end_form( );
fl_deactivate_object( yes )
fl_set_object_color( yes, FL_INACTIVE_COL );
fl_show_form( form, FL_PLACE_MOUSE,
FL_NOBORDER, NULL );
fl_do_forms( );
fl_activate_object( yes );
fl_set_object_color( yes, FL_TOMATO );
fl_do_forms( );
return 0;
}
void fl_set_object_color( FL_OBJECT* aThing, color1, color2 );
Item | Color1 | Color2 |
box | Box color | not used |
text | Text color | not used |
buttons | color when released | color when pushed |
light buttons | light off | light on |
bitmap button | box color | bitmap color |
slider | background color | slider color |
input | not selected | when selected |
Some Colors
FL_RED
FL_BLACK
FL_GREEN
FL_YELLOW
FL_BLUE
FL_CYAN
FL_MAGENTA
FL_WHITE
FL_TOMATO
FL_SLATEBLUE
FL_ORCHID
FL_DARKGOLD
void fl_set_object_bottype( FL_OBJECT* aThing, int boxtype );
boxtype
- FL_UP_BOX
- Comes out of the screen
- FL_DOWN_BOX
- Goes down into the screen
- FL_FLAT_BOX
- Flat box with no border
- FL_BORDER_BOX
- Flat box with border
- FL_FRAME_BOX
- Flat box with engraved border
- FL_SHADOW_BOX
- Flat box with a shadow
- FL_ROUNDED_BOX
- Rounded box
- FL_RFLAT_BOX
- Rounded box without border
- FL_RSHADOW_BOX
- Rounded box with shadow
- FL_OVAL_BOX
- Box shaped like an ellipse
- FL_NO_BOX
- No box at all
Label Color
void fl_set_object_lcol( FL_OBJECT* aThing, FL_COLOR color );
Label Size
void fl_set_object_lsize( FL_OBJECT* aThing, int LabelSize );
FL_TINY_SIZE | 8pt |
FL_SMALL_SIZE | 10pt |
FL_NORMAL_SIZE | 12pt |
FL_MEDUIM_SIZE | 14pt |
FL_LARGE_SIZE | 18pt |
FL_HUGE_SIZE | 24pt |
Font Style
void fl_set_object_lstyle( FL_OBJECT* aThing, int FontStyle );
FL_NORMAL_STYLE | Normal text |
FL_BOLD_STYLE | Boldface |
FL_ITALIC_STYLE | Italic |
FL_BOLDITALIC_STYLE | Bold Italic |
FL_FIXED_STYLE | Fixed width |
FL_FIXEDBOLD_STYLE |
FL_FIXEDITALIC_STYLE |
FL_FIXEDBOLDITALIC_STYLE |
FL_TIMES_STYLE | Times-Roman |
FL_TIMESBOLD_STYLE |
FL_TIMESITALIC_STYLE |
FL_TIMESBOLDITALIC_STYLE |
FL_SHADOW_STYLE |
FL_ENGRAVED_STYLE |
FL_EMBOSSED_STYLE |
#include "forms.h"
int main( int argc, char *argv[] )
{
int answer;
int NumberOfButtons; // range 1, 2, 3
char* UserText ;
fl_show_message("Line one of messge", "Line two of messge",
"Line 3 of messge");
const int CENTERED = 1;
const int NOTCENTERED = 0;
fl_show_alert("Line one of messge", "Line two of messge",
"Line 3 of messge", CENTERED );
answer = fl_show_question("Answer = 1 if user selects yes",
"Answer = 0 if user selects no", "");
NumberOfButtons = 2;
answer = fl_show_question("Line 1", "Line 2", "Line3",
NumberOfButtons , "Button label 1",
"Button label 2", "" );
UserText = fl_show_input("The question", "Default Answer" );
return 1;
}
void yesButtonPressed( FL_OBJECT* theButton, long NotUsed) {
cout << "The no button was pressed" << endl;
exit(0);
};
void noButtonPressed( FL_OBJECT* theButton, long color) {
cout << "The yes button was pressed" << endl;
fl_set_object_color( theButton, color, color);
};
int main( int argc, char *argv[] )
{
FL_FORM *form;
FL_OBJECT *yes, *no, *button;
fl_initialize( argv[0], "FormDemo", 0, 0 , &argc, argv );
form = fl_bgn_form( FL_UP_BOX, 320, 120 );
fl_add_box( FL_NO_BOX, 160, 80, 0, 0, "Quit?" );
yes = fl_add_button(FL_NORMAL_BUTTON,
40, 20, 80, 30, "Yes" );
fl_set_object_callback( yes, yesButtonPressed,
(long) FL_RED);
no = fl_add_button( FL_NORMAL_BUTTON,
200, 20, 80, 30, "No" );
fl_set_object_callback( no, noButtonPressed, 0);
fl_end_form( );
fl_show_form( form, FL_PLACE_MOUSE,
FL_NOBORDER, NULL );
fl_do_forms( );
return 0;
}
#include <iostream.h>
#include <string.h>
#include "forms.h"
class Counter {
public:
void increase() { count++;};
void decrease() { count--;};
Counter() {count = 0;};
int getCount() { return count;};
private:
int count;
};
void decreaseButtonPressed( FL_OBJECT* button, long NotUsed) {
Counter* CurrentCount = (Counter*) counterPointer;
MyCount ->decrease();
cout << "The count is" << CurrentCount ->getCount() << endl;
};
void increaseButtonPressed( FL_OBJECT* bttn, long counterPointer) {
Counter* CurrentCount = (Counter*) counterPointer;
MyCount ->increase();
cout << "The count is" << CurrentCount ->getCount() << endl;
};
int main( int argc, char *argv[] )
{
FL_FORM *form;
FL_OBJECT *increase, *decrease;
Counter TheCount;
fl_initialize( argv[0], "FormDemo", 0, 0 , &argc, argv );
form = fl_bgn_form( FL_UP_BOX, 320, 120 );
fl_add_box( FL_NO_BOX, 160, 80, 0, 0,
"Do you want to Quit?" );
increase = fl_add_button( FL_NORMAL_BUTTON,
40, 20, 80, 30, "Increase" );
fl_set_object_callback( increase, increaseButtonPressed,
(long) &TheCount );
decrease = fl_add_button( FL_NORMAL_BUTTON,
200, 20, 80, 30, "Decrease" );
fl_set_object_callback( decrease, decreaseButtonPressed,
(long) &TheCount );
fl_end_form( );
fl_show_form( form, FL_PLACE_MOUSE,
FL_NOBORDER, NULL );
fl_do_forms( );
return 0;
}