CS535 Object-Oriented Programming & Design
Fall Semester, 1996
Doc 27 AWT Layouts, Panels, Canvases
[To Lecture Notes Index]
San Diego State University -- This page last updated Nov 21, 1996

Contents of Doc 27 AWT Layouts, Panels, Canvases
- References
- Layouts
- FlowLayout
- GridLayout
- BorderLayout
- Panels
- Canvas
- CardLayout
- GridBagLayout
Core Java, Chapter 7
Graphic Java, Geary and McClellan, Chapter 7
Need to specify where items will appear in a window that changes size
Layouts provide a flexible way to place items in a window without specifying
coordinates
Layouts determine place components will be seen on the screen
A component can be:
Button | Label | Scrollbar |
Canvas | List | TextArea |
Checkbox | Panel | TextField |
About LayoutsBorderLayout
Divides its components into regions
CardLayout
CardLayout contains cards
Each card contains one component
One card can be shown at a time
FlowLayout
Displays components left to right, top to bottom
GridLayout
Displays components in a grid of rows and columns
GridBagLayout
You can do almost anything with this once you understand it
Constructors
FlowLayout()
- Constructs a new Flow Layout with a centered alignment.
FlowLayout( int alignment )
- Constructs a new Flow Layout with the specified alignment.
FlowLayout(int alignment , int horizontalGap, int verticalGap )
- Constructs a new Flow Layout with the specified alignment and gap values.
Constants for Alignment
CENTER LEFT RIGHT
Center is default
Methods
addLayoutComponent(String,Component)
layoutContainer(Container)
minimumLayoutSize(Container)
preferredLayoutSize(Container)
removeLayoutComponent(Component)
toString()
How A FlowLayout Works
import java.awt.*;
class FlowExample extends Frame {
public FlowExample( int width, int height ) {
setTitle( "Flow Example" );
resize( width, height );
setLayout( new FlowLayout( FlowLayout.LEFT) );
for ( int label = 1; label < 10; label++ )
add( new Button( String.valueOf( label ) ) );
show();
}
public static void main( String args[] ) {
new FlowExample( 175, 100 );
new FlowExample( 175, 100 );
}
}
Alignment Examples
import java.awt.*;
class AlignmentExample extends Frame {
public AlignmentExample( int width, int height,
String title, int alignment ) {
setTitle( title );
resize( width, height );
setLayout( new FlowLayout( alignment) );
add( new Button( "one") );
add( new Button( "two") );
show();
}
public static void main( String args[] ) {
new AlignmentExample( 150, 50, "Left", FlowLayout.LEFT);
new AlignmentExample( 150, 50, "Right", FlowLayout.RIGHT);
new AlignmentExample( 150, 50, "Center", FlowLayout.CENTER);
}
}
Gap Example
class GapExample extends Frame {
public GapExample( int width, int height, String title,
int HorizontalGap, int VerticalGap ) {
setTitle( title );
resize( width, height );
setLayout( new FlowLayout( FlowLayout.LEFT,
HorizontalGap,VerticalGap) );
for ( int label = 1; label < 10; label++ )
add( new Button( String.valueOf( label ) ) );
show();
}
public static void main( String args[] ) {
new GapExample( 175, 100, "Small Gap", 2, 2 );
new GapExample( 175, 100, "Large Gap", 10, 20 );
}
}
Constructors
GridLayout(int, int)
- Creates a grid layout with the specified rows and columns.
GridLayout( int rows, cols, horizontalGap, verticalGap )
- Creates a grid layout with the specified rows, columns, horizontal gap, and
vertical gap.
Methods
addLayoutComponent(String,Component)
layoutContainer(Container)
minimumLayoutSize(Container)
preferredLayoutSize(Container)
removeLayoutComponent(Component)
toString()
A layout manager for a container that lays out grids
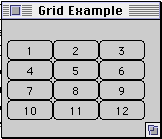
Grid Example
import java.awt.*;
class GridLayoutExample extends Frame {
public GridLayoutExample( int widthInPixels, int heightInPixels ) {
setTitle( "Grid Example" );
resize( widthInPixels, heightInPixels );
int numberOfRows = 4;
int numberOfColumns = 3;
setLayout( new GridLayout( numberOfRows,
numberOfColumns ) );
for ( int label = 1; label < 13; label++ )
add( new Button( String.valueOf( label ) ) );
show();
}
public static void main( String args[] ) {
new GridLayoutExample( 175, 100 );
}
}
addLayoutComponent(String,Component)
layoutContainer(Container)
minimumLayoutSize(Container)
preferredLayoutSize(Container)
removeLayoutComponent(Component)
toString()
The "North", "South", "East" and "West" components get placed and sized
according to their preferred sizes and the constraints of the container's size.
The "Center" component will get any space left over.
The default layout for a frame is BorderLayout.
BorderLayout
import java.awt.*;
class BorderLayoutExample extends Frame {
public BorderLayoutExample( int widthInPixels,
int heightInPixels ) {
setTitle( "BorderLayout Example" );
resize( widthInPixels, heightInPixels );
setLayout( new BorderLayout() );
add( "North", new Button( "North" ) );
add( "Center", new Button( "Center" ) );
add( "East", new Scrollbar( Scrollbar.VERTICAL ) );
add( "South", new Scrollbar( Scrollbar.HORIZONTAL ) );
show();
}
public static void main( String args[] ) {
new BorderLayoutExample( 175, 100 );
}
}
Panels allow use of one layout inside of another
A panel can contain layouts
Mixing layouts increases the flexibility of layouts
The default layout for all panels is FlowLayout
import java.awt.*;
class PanelExample extends Frame {
public PanelExample( int widthInPixels, int heightInPixels ) {
setTitle( "Panel Example" );
resize( widthInPixels, heightInPixels );
setLayout( new BorderLayout() );
Panel buttonDisplay = new Panel();
buttonDisplay.setLayout( new FlowLayout() );
for ( int label = 1; label < 6; label++ )
buttonDisplay.add( new Button( String.valueOf( label )) );
add( "North", buttonDisplay );
add( "Center", new Button( "Center" ) );
add( "East", new Scrollbar( Scrollbar.VERTICAL ) );
add( "South", new Scrollbar( Scrollbar.HORIZONTAL ) );
show();
}
public static void main( String args[] ) {
new PanelExample( 175, 100 );
}
}
Result of Panel Example
Note that the buttons do not wrap to second row of buttons event though they
are in a FlowLayout!
The FlowLayout is in north part of a BorderLayout
Only one row is available!
Problem with Paint
class DrawExample extends Frame {
public DrawExample( int width, int height ) {
setTitle( "Draw Problem" );
resize( width, height );
setLayout( new FlowLayout( FlowLayout.LEFT, 20, 20 ) );
for ( int label = 1; label < 10; label++ )
add( new Button( String.valueOf( label ) ) );
show();
}
public void paint( Graphics screen ) {
screen.drawString( "This is a mess", 20 , 30 );
}
public static void main( String args[] ) {
new DrawExample( 175, 100 );
}
}
A
canvas is a rectangular region which you can draw on.
Need to subclass the Canvas class to use it.
Canvas Methods
addNotify()
- Creates the peer of the canvas.
paint(Graphics)
- Paints the canvas in the default background color.
Sample Canvas
class SimpleCanvas extends Canvas{
public void paint( Graphics drawOnMe ){
int startX = 0;
int startY = 0;
int endX = 30;
int endY = 30;
drawOnMe.drawLine( startX, startY, endX, endY );
int width = 5;
int height = 5;
drawOnMe.drawOval( endX, endY, width, height );
}
}
Using the Canvas
class CanvasExample extends Frame {
public CanvasExample( int widthInPixels, int heightInPixels ) {
setTitle( "Canvas Example" );
resize( widthInPixels, heightInPixels );
setLayout( new BorderLayout() );
Panel buttonDisplay = new Panel();
buttonDisplay.setLayout( new FlowLayout() );
for ( int label = 1; label < 6; label++ )
buttonDisplay.add( new Button( String.valueOf( label ) ) );
add( "North", buttonDisplay );
add( "Center", new SimpleCanvas() );
add( "East", new Scrollbar( Scrollbar.VERTICAL ) );
add( "South", new Scrollbar( Scrollbar.HORIZONTAL ) );
show();
}
public static void main( String args[] ) {
new CanvasExample( 175, 150 );
}
}
What Going On Here?
class CanvasProblem extends Frame {
public CanvasProblem( int widthInPixels, int heightInPixels ) {
setTitle( "Canvas Problem" );
resize( widthInPixels, heightInPixels );
setLayout( new BorderLayout() );
Panel buttonDisplay = new Panel();
buttonDisplay.setLayout( new FlowLayout() );
for ( int label = 1; label < 6; label++ )
buttonDisplay.add( new Button( String.valueOf( label ) ) );
add( "Center", buttonDisplay );
add( "North", new SimpleCanvas() );
add( "East", new Scrollbar( Scrollbar.VERTICAL ) );
add( "South", new Scrollbar( Scrollbar.HORIZONTAL ) );
show();
}
public static void main( String args[] ) {
new CanvasProblem( 175, 150 );
}
}
Preferred/Minimum Sizes
Components have a preferred size and a minimum size
Canvas default preferred size is 0 width , 0 height
BorderLayout
- North, South respect preferred height, ignores preferred width
-
- East, West respect preferred width, ignores preferred height
-
- Center ignores preferred height and preferred width
CardLayout ignores preferred height and preferred width
FlowLayout respects preferred height and preferred width
GridLayout ignores preferred height and preferred width
GridBagLayout varies on GridBagConstrants for component
Override preferredSize/minimumSizein Canvas subclasses
class SimpleCanvas extends Canvas{
Dimension preferredSize;
public SimpleCanvas( int width, int height ) {
preferredSize = new Dimension( width, height );
}
public void paint( Graphics drawOnMe ){
int startX = 0;
int startY = 0;
int endX = 30;
int endY = 30;
drawOnMe.drawLine( startX, startY, endX, endY );
int width = 5;
int height = 5;
drawOnMe.drawOval( endX, endY, width, height );
}
public Dimension preferredSize() {
return preferredSize;
}
public Dimension minimumSize() {
return new Dimension( 5, 5 );
}
}
SimpleCanvas Fixed
class CanvasProblem extends Frame {
public CanvasProblem( int widthInPixels, int heightInPixels ) {
setTitle( "Canvas Fixed" );
resize( widthInPixels, heightInPixels );
setLayout( new BorderLayout() );
Panel buttonDisplay = new Panel();
buttonDisplay.setLayout( new FlowLayout() );
for ( int label = 1; label < 6; label++ )
buttonDisplay.add( new Button( String.valueOf( label ) ) );
add( "Center", buttonDisplay );
add( "North", new SimpleCanvas( 20, 30 ) );
add( "East", new Scrollbar( Scrollbar.VERTICAL ) );
add( "South", new Scrollbar( Scrollbar.HORIZONTAL ) );
show();
}
public static void main( String args[] ) {
new CanvasProblem( 175, 150 );
}
}
Methods
addLayoutComponent(String,Component)
first(Container)
last(Container)
layoutContainer(Container)
minimumLayoutSize(Container)
next(Container)
preferredLayoutSize(Container)
previous(Container)
removeLayoutComponent(Component)
show(Container,String)
toString()
A layout manager for a container that contains several 'cards'. Only one card
is visible at a time, allowing you to flip through the cards.
Example
import java.awt.*;
class CardLayoutExample extends Frame {
CardLayout threeCards;
Panel deck;
public CardLayoutExample( int widthInPixels, int heightInPixels )
{
setTitle( "CardLayoutExample Example" );
resize( widthInPixels, heightInPixels );
setLayout( new BorderLayout() );
threeCards = new CardLayout();
deck = new Panel();
deck.setLayout( threeCards );
deck.add( "Ace", new Button( "Ace" ) );
deck.add( "King", new Button( "King" ) );
deck.add( "Queen", new Button( "Queen" ) );
add( "Center", deck );
add( "North", new Button( "Next" ) );
show();
}
public boolean action( Event processNow, Object buttonPressed ) {
if ( buttonPressed.equals( "Next" ) )
threeCards.next( deck );
else return super.action( processNow, buttonPressed );
return true;
}
public static void main( String args[] ) {
new CardLayoutExample(200, 200);
}
}
GridBagConstrants
anchor | HORIZONTAL | REMAINDER |
BOTH | insets | SOUTH |
CENTER | ipadx | SOUTHEAST |
EAST | ipady | SOUTHWEST |
fill | NONE | VERTICAL |
gridheight | NORTH | weightx |
gridwidth | NORTHEAST | weighty |
gridx | NORTHWEST | WEST | |
gridy | RELATIVE | |
addLayoutComponent(String,Component)
AdjustForGravity(GridBagConstraints,Rectangle)
ArrangeGrid(Container)
getConstraints(Component)
getLayoutDimensions()
GetLayoutInfo(Container,int)
getLayoutOrigin()
getLayoutWeights()
GetMinSize(Container,GridBagLayoutInfo)
layoutContainer(Container)
location(int,int)
lookupConstraints(Component)
minimumLayoutSize(Container)
preferredLayoutSize(Container)
removeLayoutComponent(Component)
setConstraints(Component,GridBagConstraints)
toString()