CS535 Object-Oriented Programming & Design
Fall Semester, 1996
Doc 25 AWT Lists, Menus, Text
[To Lecture Notes Index]
San Diego State University -- This page last updated Nov 19, 1996

Contents of Doc 25 AWT Lists, Menus, Text
- References
- Checkbox
- Checkbox Groups - Radio buttons
- Choice Boxes - Drop-down Lists
- List Box
- Text Area
- TextField
- Label
- Menu
Core Java, Chapter 7
import java.awt.*;
class CheckboxExample extends Frame {
private Checkbox left = new Checkbox( "Left" );
private Checkbox right = new Checkbox( "Right" );
public CheckboxExample( int widthInPixels, int heightInPixels ) {
setTitle( "Checkbox Example" );
resize( widthInPixels, heightInPixels );
setLayout( new FlowLayout() );
add( left );
add( right );
show();
}
public boolean action( Event processNow, Object checkBoxState ) {
if ( processNow.target.equals( left ) )
reportChange( left );
else if ( processNow.target.equals( right ) )
reportChange( right );
else return super.action( processNow, checkBoxState );
return true;
}
private void reportChange( Checkbox selectedBox ) {
System.out.print( selectedBox.getLabel() + " box changed " );
System.out.println( "value is " + selectedBox.getState() );
}
public static void main( String args[] ) {
new CheckboxExample(200, 50);
}
}
Output
Checkbox Constructors
Checkbox()
- Constructs a Checkbox with no label, no Checkbox group, and initialized to
a false state.
Checkbox(String)
- Constructs a Checkbox with the specified label, no Checkbox group, and
initialized to a false state.
Checkbox(String, CheckboxGroup, boolean)
- Constructs a Checkbox with the specified label, specified Checkbox group,
and specified boolean state.
Checkbox Methods
getCheckboxGroup()
getLabel()
getState()
setCheckboxGroup(CheckboxGroup)
setLabel(String)
setState(boolean)
Changing Checkbox label
import java.awt.*;
class CheckboxExample extends Frame {
private Checkbox toggle = new Checkbox( "Off");
public CheckboxExample( int widthInPixels, int heightInPixels ) {
setTitle( "Checkbox Example" );
resize( widthInPixels, heightInPixels );
setLayout( new FlowLayout() );
add( toggle );
show();
}
public boolean action( Event processNow, Object checkBoxState ) {
if ( processNow.target.equals( toggle ) )
changeLabel( toggle );
else return super.action( processNow, checkBoxState );
return true;
}
private void changeLabel( Checkbox selectedBox ) {
if ( selectedBox.getState() == true )
selectedBox.setLabel( "On" );
else if ( selectedBox.getState() == false )
selectedBox.setLabel( "Off" );
}
public static void main( String args[] ){
new CheckboxExample(200, 50);
}
}
Output
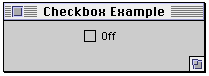
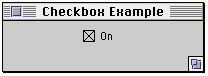
import java.awt.*;
class RadioButton extends Frame {
private CheckboxGroup directions = new CheckboxGroup();
private Checkbox left = new Checkbox( "Left", directions, false);
private Checkbox right = new Checkbox( "Right", directions, true);
public RadioButton( int widthInPixels, int heightInPixels ) {
setTitle( "Radio Button Example" );
resize( widthInPixels, heightInPixels );
setLayout( new FlowLayout() );
add( left );
add( right );
show();
}
public boolean action( Event processNow, Object checkBoxState) {
if ( processNow.target.equals( left ) )
reportChange( left );
else if ( processNow.target.equals( right ) )
reportChange( right );
else return super.action( processNow, checkBoxState);
return true;
}
private void reportChange( Checkbox selectedBox ) {
System.out.print( selectedBox.getLabel() + " box changed " );
System.out.println( "value is " + selectedBox.getState() );
}
public static void main( String args[] ) {
new RadioButton(200, 50);
}
}
Output
CheckboxGroup Methods
getCurrent() | setCurrent(Checkbox) |
import java.awt.*;
class ChoiceBoxes extends Frame {
Choice directions = new Choice();
public ChoiceBoxes( int widthInPixels, int heightInPixels ) {
setTitle( "ChoiceBox Example" );
resize( widthInPixels, heightInPixels );
setLayout( new FlowLayout() );
directions.addItem( "Left" );
directions.addItem( "Right" );
directions.addItem( "Up" );
directions.addItem( "Down" );
add( directions );
show();
}
public boolean action( Event processNow, Object textSelected ) {
if ( processNow.target == directions )
System.out.println( "You selected " +
( String ) textSelected );
else return super.action( processNow, textSelected );
return true;
}
public static void main( String args[] ) {
new ChoiceBoxes(200, 200);
}
}
Output
Choice Methods
addItem(String) | getItem(int) | paramString() |
addNotify() | getSelectedIndex() | select(int) |
countItems() | getSelectedItem() | select(String) |
import java.awt.*;
class ListExample extends Frame {
List directions ;
public ListExample( int widthInPixels, int heightInPixels ) {
setTitle( "List Box Example" );
resize( widthInPixels, heightInPixels );
setLayout( new FlowLayout() );
boolean areMultipleSelectionsAllowed = false;
int numberDisplayed = 4;
directions = new List( numberDisplayed,
areMultipleSelectionsAllowed );
directions.addItem( "Left" );
directions.addItem( "Right" );
directions.addItem( "Up" );
directions.addItem( "Down" );
add( directions );
show();
}
public boolean action( Event processNow, Object textSelected ) {
if ( processNow.target.equals( directions ) )
System.out.println( "You selected " +
( String ) textSelected );
else return super.action( processNow, textSelected );
return true;
}
public static void main( String args[] ) {
new ListExample(200, 100);
}
}
Output
List Constructors
List()
- Creates a new scrolling list initialized with no visible Lines or multiple
selections.
List(int, boolean)
- Creates a new scrolling list initialized with the specified number of
visible lines and a boolean stating whether multiple selections are allowed or
not.
List Methods
addItem(String) | getSelectedItems() |
addItem(String,int) | getVisibleIndex() |
addNotify() | isSelected(int) |
allowsMultipleSelections() | makeVisible(int) |
clear() | minimumSize() |
countItems() | minimumSize(int) |
delItem(int) | paramString() |
delItems(int,int) | preferredSize() |
deselect(int) | preferredSize(int) |
getItem(int) | removeNotify() |
getRows() | replaceItem(String,int) |
getSelectedIndex() | select(int) |
getSelectedIndexes() | setMultipleSelections(boolean) |
getSelectedItem() | |
import java.awt.*;
class TextAreas extends Frame {
TextArea userInput;
Button done = new Button( "Done Typing" );
public TextAreas( int widthInPixels, int heightInPixels ) {
setTitle( "Text Area Example" );
resize( widthInPixels, heightInPixels );
setLayout( new FlowLayout() );
int numberOfLines = 3;
int numberOfColumns = 30;
userInput = new TextArea( numberOfLines, numberOfColumns );
add( userInput );
add( done );
show();
}
public boolean action( Event processNow, Object buttonLabel ) {
if ( processNow.target == done )
checkUserInput();
else return super.action( processNow, buttonLabel );
return true;
}
private void checkUserInput() {
System.out.println( "You typed: " + userInput.getText() );
System.out.println( "Selected text>" +
userInput.getSelectedText() +"<" );
userInput.setText( "Hi Mom");
}
public static void main( String args[] ) {
new TextAreas(300, 150);
}
}
Output
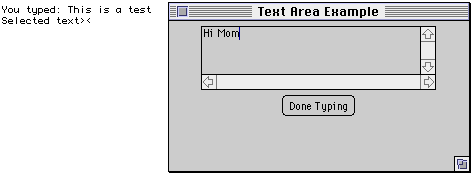
TextArea Constructors
TextArea()
- Constructs a new TextArea.
-
TextArea(int, int)
- Constructs a new TextArea with the specified number of rows and columns.
TextArea(String)
- Constructs a new TextArea with the specified text displayed.
TextArea(String, int, int)
- Constructs a new TextArea with the specified text and number of rows and
columns.
TextArea Methods
addNotify() | minimumSize(int,int) |
appendText(String) | paramString() |
getColumns() | preferredSize() |
getRows() | preferredSize(int,int) |
insertText(String,int) | replaceText(String,int,int) |
minimumSize() | |
How can I tell when the user is done Typing?
class TextEvents extends TextArea {
public TextEvents( int numberOfLines, int numberOfColumns ) {
super( numberOfLines, numberOfColumns );
}
public boolean handleEvent( Event userAction ) {
if ( userAction.id == Event.ACTION_EVENT )
System.out.println( "ACTION_EVENT" );
else if ( userAction.id == Event.DOWN )
System.out.println( "DOWN" );
else if ( userAction.id == Event.GOT_FOCUS )
System.out.println( "GOT_FOCUS" );
else if ( userAction.id == Event.KEY_ACTION )
System.out.println( "KEY_ACTION" );
else if ( userAction.id == Event.KEY_ACTION_RELEASE )
System.out.println( "KEY_ACTION_RELEASE" );
else if ( userAction.id == Event.KEY_PRESS )
System.out.println( "KEY_PRESS" );
else if ( userAction.id == Event.KEY_RELEASE )
System.out.println( "KEY_RELEASE" );
else if ( userAction.id == Event.LOST_FOCUS )
System.out.println( "LOST_FOCUS" );
else if ( userAction.id == Event.MOUSE_DOWN )
System.out.println( "MOUSE_DOWN" );
else if ( userAction.id == Event.MOUSE_DRAG )
System.out.println( "MOUSE_DRAG" );
else if ( userAction.id == Event.MOUSE_ENTER )
System.out.println( "MOUSE_ENTER" );
else if ( userAction.id == Event.MOUSE_EXIT )
System.out.println( "MOUSE_EXIT" );
else if ( userAction.id == Event.MOUSE_MOVE )
System.out.println( "MOUSE_MOVE" );
else if ( userAction.id == Event.MOUSE_UP )
System.out.println( "MOUSE_UP" );
return super.handleEvent( userAction );
}
Result of "Is User Done Typing?"
class TextAreas extends Frame {
public TextAreas( int widthInPixels, int heightInPixels ) {
setTitle( "Text Area Example" );
resize( widthInPixels, heightInPixels );
setLayout( new FlowLayout() );
add( new TextEvents( 3, 30 ) );
show();
}
public static void main( String args[] ) {
new TextAreas(300, 150);
}
}
import java.awt.*;
class TextExample extends Frame {
TextField userInput;
public TextExample( int widthInPixels, int heightInPixels ) {
setTitle( "Text Field Example" );
resize( widthInPixels, heightInPixels );
setLayout( new FlowLayout() );
String initialText = "Hi Dad";
int numberOfColumns = 30;
userInput = new TextField( initialText, numberOfColumns );
add( userInput );
add( new Button( "Done Typing" ) );
show();
}
public boolean action( Event processNow, Object buttonPressed ) {
if ( buttonPressed.equals( "Done Typing" ) )
checkUserInput();
else return super.action( processNow, buttonPressed );
return true;
}
private void checkUserInput() {
System.out.println( "You typed: " + userInput.getText() );
System.out.println( "Selected text>" +
userInput.getSelectedText() +"<" );
userInput.setText( "Hi Mom");
userInput.setEchoCharacter( 'X' );
}
public static void main( String args[] ) {
new TextExample( 300, 200 );
}
}
TextField Constructors
TextField()
- Constructs a new TextField.
TextField(int)
- Constructs a new TextField initialized with the specified columns.
TextField(String)
- Constructs a new TextField initialized with the specified text.
TextField(String, int)
- Constructs a new TextField initialized with the specified text and columns.
TextField Methods
addNotify() | minimumSize(int) |
echoCharIsSet() | paramString() |
getColumns() | preferredSize() |
getEchoChar() | preferredSize(int) |
minimumSize() | setEchoCharacter(char) |
Is the User Done Typing?
class TextEvents extends TextField {
public TextEvents( String text, int numberOfColumns ) {
super( text, numberOfColumns );
}
public boolean handleEvent( Event userAction ) {
if ( userAction.id == Event.ACTION_EVENT )
System.out.println( "ACTION_EVENT" );
else if ( userAction.id == Event.DOWN )
System.out.println( "DOWN" );
else if ( userAction.id == Event.GOT_FOCUS )
System.out.println( "GOT_FOCUS" );
else if ( userAction.id == Event.KEY_ACTION )
System.out.println( "KEY_ACTION" );
else if ( userAction.id == Event.KEY_ACTION_RELEASE )
System.out.println( "KEY_ACTION_RELEASE" );
else if ( userAction.id == Event.KEY_PRESS )
System.out.println( "KEY_PRESS" );
else if ( userAction.id == Event.KEY_RELEASE )
System.out.println( "KEY_RELEASE" );
else if ( userAction.id == Event.LOST_FOCUS )
System.out.println( "LOST_FOCUS" );
else if ( userAction.id == Event.MOUSE_DOWN )
System.out.println( "MOUSE_DOWN" );
else if ( userAction.id == Event.MOUSE_DRAG )
System.out.println( "MOUSE_DRAG" );
else if ( userAction.id == Event.MOUSE_ENTER )
System.out.println( "MOUSE_ENTER" );
else if ( userAction.id == Event.MOUSE_EXIT )
System.out.println( "MOUSE_EXIT" );
else if ( userAction.id == Event.MOUSE_MOVE )
System.out.println( "MOUSE_MOVE" );
else if ( userAction.id == Event.MOUSE_UP )
System.out.println( "MOUSE_UP" );
return super.handleEvent( userAction );
}
}
Result of "User Done Typing"
class TextAreas extends Frame {
public TextAreas( int widthInPixels, int heightInPixels ) {
setTitle( "Text Area Example" );
resize( widthInPixels, heightInPixels );
setLayout( new FlowLayout() );
add( new TextEvents( "a", 30 ) );
add( new TextEvents( "b", 30 ) );
show();
}
public static void main( String args[] ) {
new TextAreas(300, 150);
}
}
import java.awt.*;
class LabelExample extends Frame {
public LabelExample( int widthInPixels, int heightInPixels ) {
setTitle( "Label Example" );
resize( widthInPixels, heightInPixels );
setLayout( new FlowLayout() );
add( new Label( "Hi Mom" ) );
add( new Label( "Hi Dad", Label.CENTER ) );
add( new Label( "Good Bye", Label.RIGHT ) );
add( new Label( "Good Luck", Label.LEFT ) );
show();
}
public static void main( String args[] ) {
new LabelExample(150, 100);
}
}
Label With Text
import java.awt.*;
class TextExample extends Frame {
TextField userInput;
public TextExample( int widthInPixels, int heightInPixels ) {
setTitle( "Text Field Example" );
resize( widthInPixels, heightInPixels );
setLayout( new FlowLayout() );
add( new Label( "Type Here", Label.RIGHT ) );
int numberOfColumns = 20;
userInput = new TextField( "", numberOfColumns );
add( userInput );
add( new Button( "Done Typing" ) );
show();
}
public boolean action( Event processNow, Object buttonPressed ) {
if ( buttonPressed.equals( "Done Typing" ) )
checkUserInput();
else return super.action( processNow, buttonPressed );
return true;
}
private void checkUserInput() {
System.out.println( "You typed: " + userInput.getText() );
}
public static void main( String args[] ) {
new TextExample( 300, 200 );
}
}

Label Constructors
Label()
- Constructs an empty label.
Label(String)
- Constructs a new label with the specified String of text.
Label(String, int)
- Constructs a new label with the specified String of text and the specified
alignment.
Label Constants
Label Methods
addNotify() | paramString() |
getAlignment() | setAlignment(int) |
getText() | setText(String) |
import java.awt.*;
class MenuExample extends Frame {
public MenuExample( int widthInPixels, int heightInPixels ) {
setTitle( "Menu Example" );
MenuBar allMenus = new MenuBar();
Menu gradeMenu = new Menu( "Grade" );
gradeMenu.add( new MenuItem( "A" ) );
gradeMenu.add( new MenuItem( "B" ) );
gradeMenu.addSeparator();
gradeMenu.add( new MenuItem( "C" ) );
gradeMenu.addSeparator();
Menu failingGradeMenu = new Menu( "Bad News" );
failingGradeMenu.add( new MenuItem( "D" ) );
failingGradeMenu.add( new CheckboxMenuItem( "F" ) );
gradeMenu.add( failingGradeMenu );
allMenus.add( gradeMenu );
setMenuBar( allMenus );
show();
}
public boolean action( Event processNow, Object menuItem ) {
if ( processNow.target instanceof MenuItem )
if ( menuItem.equals( "F" ) )
System.out.println( "Trouble" );
else return super.action( processNow, menuItem );
return true;
}
public static void main( String args[] ) {
new MenuExample( 200, 100 );
}
}
Menu Classes
MenuComponent
getFont() | paramString() | setFont(Font) |
getParent() | postEvent(Event) | toString() |
getPeer() | removeNotify() | |
MenuBar
add(Menu) | getHelpMenu() | remove(MenuComponent) |
addNotify() | getMenu(int) | removeNotify() |
countMenus() | remove(int) | setHelpMenu(Menu) |
MenuItem
addNotify() | getLabel() |
disable() | isEnabled() |
enable() | paramString() |
enable(boolean) | setLabel(String) |
Menu
add(MenuItem) | getItem(int) |
add(String) | isTearOff() |
addNotify() | remove(int) |
addSeparator() | remove(MenuComponent) |
countItems() | removeNotify() |
CheckboxMenuItem
addNotify() | paramString() |
getState() | setState(boolean) |