CS 596: Client Server Programming
Design
[To Lecture Notes Index]
San Diego State University -- This page last updated April 28, 1995

Contents of DesignLecture
- Overview of Design
- Exploratory Phase
- Exploratory Phase
- Modules
- Finding Modules
- Record Your Candidate Modules
- Finding Abstract Classes
- Responsibilities
- Identifying Responsibilities
- Assigning Responsibilities
- Recording Responsibilities
- Collaboration
- Finding Collaborations
- Recording Collaborations
- What are the goals of the system?
-
- What must the system accomplish?
-
- What module are required to model the system and accomplish the
goals?
- What are their tasks, responsibilities?
- What does each module have to know in order to accomplish each goal it is
involved with?
-
- What steps toward accomplishing each goal is it responsible for?
- With whom will each module collaborate in order to accomplish each of its
responsibilities?
-
- What is the nature of the module s' collaboration
Clients Servers
Module collaboration:
- One module requests a service of another module
Client
- The module making the request
Server
- The module receiving the request
Noun phrases in requirements specification or system description
Look at these phrases. Some will be obvious modules, some will be obvious
nonsense, and some will fall between obvious and nonsense. Skip the nonsense,
keep the rest. The goal is a list of candidate modules. Some items in the
list will be eliminated, others will be added later. Finding good modules is a
skill, like finding a good functional decomposition.
- Disks
- Printers Airplanes
- Model conceptual entities that form a cohesive abstraction
- Window
- File Bank Account
- If more than one word applies to a concept select the one that is most
meaningful
Finding Modules
- Be wary of the use of adjectives
- Adjective-noun phrases may or may not indicate different modules
-
- Is selection tool different than creation tool?
-
- Is start point different from end point from point?
- A sentence is passive if the subject of the verb receives the action
-
-
- Passive:
- The music was enjoyed by us
-
- Active:
- We enjoyed the music
- Model categories of modules
-
- Categories may indicate inheritence
-
- Keep them as individual modules at this point
Finding Modules
- Model known interfaces to outside world
- User interfaces
-
- Interfaces to other programs
-
- Write a description of how people will use the system. This description is
a source of interface modules.
- Model the values of attributes, not the attributes themselves
- Height of a rectangle
- Height is an attribute of rectangle
-
- Value of height is a number
-
- Rectangle can record its height
Finding Modules
Other Views
Coad and Yourdon
Structure
"Kind of" and "part of" relationships
Other systems
External systems with which the application interacts
Devices
Devices with which the application interacts
Events remembered
Historical event that must be recorded
Roles played
Different roles users play in interacting with application
Locations
Physical locations, offices and sites important to the application
Organizational units
Ross
People
Humans who carry out some function
Farmers
Places
Areas set aside for people or things
Fields on farm
Things
Physical objects or groups of objects
Plants leaves roots
Organizations
Formally organized collections of people, resources, facilities
Union farm crew
Concepts
Principles or ideas not tangible
Events
Things that happen
Shaler & Mellor[1]
Tangible things
Cars telemetry data
Roles
Mother teacher
Events
Landing interrupt
Interactions
Loan meeting
Booch[2]
"The tangible things in problem domain, the roles they play, and the events
that may occur form the candidate classes and object of our design."
Jacobson[3]
"When a user uses the system they will perform a behaviorally related sequence
of transactions in a dialogue with the system. This sequence is a use case.
Objects are found by examining all the use cases for the system."
Abbot[4]
"In an English description of the problem, the nouns are candidates for
objects; the verbs are candidates for operations."
Finding Modules
Categories of Modules
Data Managers
- Principle responsibility is to maintain data
-
- Examples: stack, collections, sets
Data Sinks or Data Sources
- Generate data or accept data and process it further
-
- Do not hold data for long
-
- Examples: Random number generator, File IO module
View or Observer Modules
- Example: GUI modules
-
Facilitator or Helper Modules
- Maintain little or no state information
-
- Assist in execution of complex tasks
Front of Card
Module: Account |
(for future use) |
(for future use) |
(for future use)
|
(for future use)
|
Back of Card
An Account represents a customer's account in the bank's database
|
Record the module name on the front of an index card. One module per card.
Write a brief description of the overall purpose of the module . The front of
the card will be filled in with information as the design process continues.
If you prefer to use some other medium (8 1/2" by 11" sheets of paper,
computer program) do so. The goal is a tool that will enhance exploring the
model. You may find better tools. However, while learning, it is hard to find
a cheaper tool than index cards. Even when you have a fancy case tool you
might find yourself using these cards to help with designing parts of programs.
An abstract class springs from a set of classes that share a useful attribute.
Look for common attributes in classes, as described by the requirement
Grouping related classes can identify candidates for abstract classes
Name the superclass that you feel each group represents
Record the superclass names
Front of Card
Module: Soil |
Superclass name |
Subclass names |
(for future use)
|
(for future use)
|
If you can't name a group:
- list the attributes shared by classes in the group and derive the name from
those attributes
-
- Divide groups into smaller, more clearly defined groups
If you still can find a name, discard the group
Examples of Attributes
Physical vs. conceptual Mouse Network protocol
Active vs. passive Producer Product
Temporary vs. permanent Event Event queue
Generic vs. specific List Event list
Shared vs. unshared Print Queue Login Name
General Guidelines
Consider public responsibilities, not private ones
Specify what gets done, not how it gets done
Keep responsibilities in general terms
Define responsibilities at an implementation-independent level
Keep all of a class's responsibilities at the same conceptual level
Requirements specification
- Verbs indicate possible actions
-
- Information indicates modules responsibilities
The Module
- What role does the module fill in the system?
-
- Statement of purpose for module implies responsibilities
Walk-through the system
- Imagine how the system will be used
-
- What situations might occur?
-
- Scenarios of using system
Scenarios[5]
Scenario
- A sequence of events between the system and an outside agent, such as a
user, a sensor, or another program
-
- Outside agent is trying to perform some task
The collection of all possible scenarios specify all the existing ways to use
the system
Normal case scenarios
- Interactions without any unusual inputs or error conditions
Special case scenarios
- Consider omitted input sequences, maximum and minimum values, and repeated
values
Error case scenarios
- Consider user error such as invalid data and failure to respond
Scenarios
Identifying Scenarios
Read the requirement specification from user's perspective
Interview users of the system
Normal ATM Scenario
The ATM asks the user to insert a card; the user inserts a card.
The ATM accepts the card and reads its serial number.
The ATM requests the password; the user enters "1234."
The ATM verifies the serial number and password with the ATM consortium; the
consortium checks it with the user's bank and notifies the ATM of
acceptance.
The ATM asks the user to select the kind of transaction; the user selects
"withdrawal."
The ATM asks the user for the amount of cash; the user enters "$100."
The ATM verifies that the amount is within predefined policy limits and asks
the consortium to process the transaction; the consortium passes the request to
the bank, which confirms the transaction and returns the new account
balance.
The ATM dispenses cash and asks the user to take it; the user takes the
cash.
The ATM asks whether the user wants to continue; the user indicates no.
The ATM prints a receipt, ejects the card and asks the user to take them; the
user takes the receipt and the card.
The ATM asks a user to insert a card.
Special Case ATM Scenario
The ATM asks the user to insert a card; the user inserts a card.
The ATM accepts the card and reads its serial number.
The ATM requests the password; the user enters "9999."
The ATM verifies the serial number and password with the ATM consortium; the
consortium checks it with the user's bank and notifies the ATM of rejection.
The ATM indicates a bad password and asks the user to reenter it; the user hits
"cancel."
The ATM ejects the card and asks the user to take it; the user takes the
card.
The ATM asks a user to insert a card.
Scenarios
Event Trace Diagram
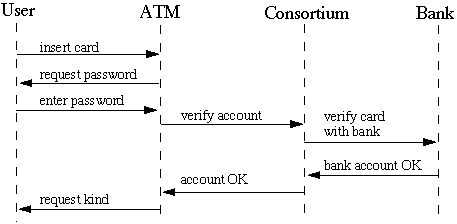
Event Flow Diagram
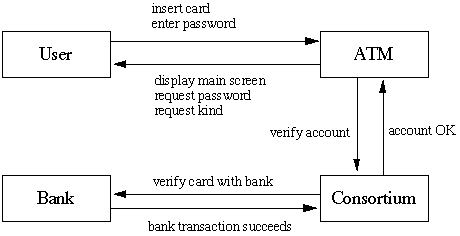
Scenarios can be visualized in event trace diagrams. Placing events from all
scenarios in an event flow diagram groups the responsibilities of entities in
the system.
Assign each responsibility to the module it logically belongs to
Evenly distribute system intelligence
Intelligence:
- What the system knows
-
- Actions that can be performed
-
- Impact on other parts of the system and users
Example: Personnel Record
- Dumb version
- A data structure holding name, age, salary, etc.
- Smart version
- An module that:
- Matches security clearance with current project
-
- Salary is in proper range
-
- Health benefits change when person gets married
Assigning Responsibilities
State responsibilities as generally as possible
Assume that each kind of drawing element knows how to draw itself. It is
better to say "drawing elements know how to draw themselves" than "a line knows
how to draw itself, a rectangle knows how to draw itself, etc."
Keep behavior with related information
Abstraction implies we should do this
Keep information about one thing in one place
If two or more module need the same information:
- Create a new module to hold the information
-
- Collapse the modules into a single module
-
- Place information in the more natural module
Assigning Responsibilities
Share responsibilities
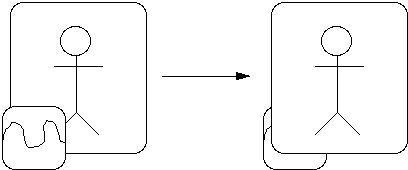
Who is responsible for updating screen when window moves?
Assigning Responsibilities
Examining Relationships Between Modules
Is-kind-of or is-a
- Implies inheritance
is-analogous-to
- If module X is-analogous-to module Y then look for superclass
is-part-of or has-a
- If module A is-part-of module B then there is no inheritance
-
- Some negotiation between A and B for responsibilities may be needed
-
- Example:
- Assume A contains a list that B uses
-
- Who sorts the list? A or B?
Common Difficulties
Missing modules
- A set of unassigned responsibilities may indicate a need for another module
-
- Group related unassigned responsibilities into a new module
Arbitrary assignment
- Sometimes a responsibility may seem to fit into two or more module
-
- Perform a walk-through the system with each choice
-
- Ask others
-
- Explore ramifications of each choice
-
- If the requirements change then which choice seems better?
Relations
or
The Data Base Problem
Mr. White works for the All-Smart Institute
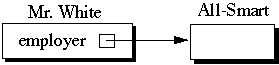
The All-Smart Institute employs Mr. White
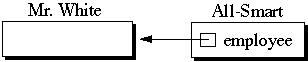
Sally is John's sister
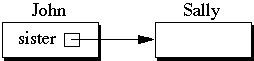
John is Sally's brother
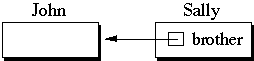
Relations
or
The Data Base Problem
Model[6]
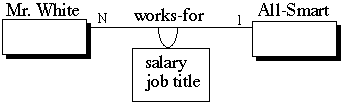
Implementation

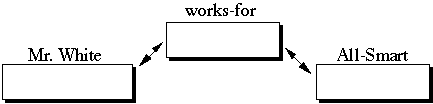
Front of Card
Module: Soil |
Superclass name |
Subclass names |
List responsibilities here
|
(for future use)
|
Represents requests from a client to a server in fulfillment of a client
responsibility
Interaction between modules
Examine module responsibilities for dependencies
For each responsibility:
- Is module capable of fulfilling this responsibility?
-
- If not, what does it need?
-
- From what other module can it acquire what it needs?
For each module :
- What does this module do or know?
-
- What other modules need the result or information?
-
- If a module has no interactions, discard it
Finding Collaborations
Examine scenarios
- Interactions in the scenarios indicate collaboration
Common Collaboration Types
The is-part-of relationship
- X is composed of Y's
-
- Composite modules
- Drawing is composed of drawing elements
-
- Some distribution of responsibilities required
- Container modules
- Arrays, lists, sets, hash tables, etc.
-
- Some have no interaction with elements
The has-knowledge-of relationship
The depends-upon relationship
Front of Card
Module: Drawing |
Superclass name |
Subclass names |
Know which elements it contains
Maintain ordering between elements
|
Drawing element
|
FootNotes
1[Shlaer, 1988], page 15
2[Booch, 1991]
3[Jacobson, 1992]
4[Abbott,1983]
5Scenarios play a role in other methodologies. See for example
[Jacobson, 1992], [Lorenz, 1993] and [Rumbaugh, 1991]. Jacobson and Lorenz use
the term "use case" instead of "scenarios".
6[Rumbaugh, 1991] discusses in great detail the problem of modeling
these types of relations between objects and classes. Also see [de Champeaux,
1993].