[To Lecture Notes Index]
San Diego State University -- This page last updated February 12, 1995

- References for Lecture
- Review of Berkeley Sockets
- Implementations
- Types of networking API
- UNIX I/O
- Berkeley Sockets Interface
- Building a TCP connection
- Berkeley socket address format
- Berkeley Socket calls
- Building a Berkeley socket connection
- Building a Berkeley TCP connection (cont.)
Comer Text Chapters 4 & 5
Why do we need/want an API (Application Programming Interface)
- To ensure adhearance to protocol specifications
- To reduce the complexity of applications.
TCP does NOT define an API. It suggests certain functionality:
- Allocate local resources for communication
- Specify local and remote communication endpoints
- Initiate a connection (client side)
- Wait for an incoming connection (server side)
- Send or receive data
- Determine when data arrives
- Generate urgent data
- Handle incoming urgent data
- Terminate a connection gracefully
- Handle connection termination from the remote side
- Abort communication
- Handle error conditions or a connection abort
- Release local resources when communcation finished
* Berkeley sockets
- 4.1c BSD (1981)
* AT&T TLI (Transport Layer Interface)
- SYSV R3.0 (1986)
Others
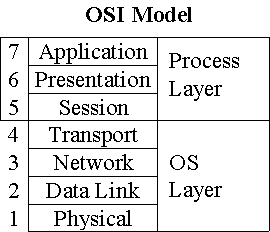
There are two basic strategies to building an interface:
1. Set of function calls when are unique to the API
- Advantage: Easy to port the API to other Oss
-
- Disadvantage: Hard to mix file I/O and network I/O
2. Overload some existing calls with network operations
- Advantage: Allows for "Object Oriented" approach
-
- Disadvantage: API tied in closely to the OS
Standard I/O calls:
- open
- close *
- read *
- write *
- lseek
- ioctl *
Berkeley sockets overload the calls marked with *
Besides these calls, others are needed to perform network specific operations
like
- Building a connection
- Sending urgent data
- etc.
Protocol independent (flexible --> complex)
Some possible protocols which can use sockets:
A connection consists of two endpoints.
An endpoint has an IP address and a Port number.
A client has one endpoint and a server has the other.
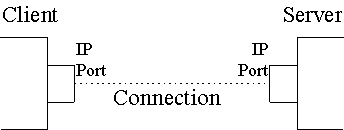
Client:
- Assigns server IP address and port number
- Lets the OS create a random port number for itself.
- Connects to the server
Server:
- Assigns well known port number to itself
- Tells OS that it wants incoming connections
- Waits for a connection
A generic address is descibed in the sockaddr structure
struct sockaddr
{
u_short sa_family; /* type of address */
char sa_data[14]; /* value of address */
};
We will use the AF_INET address family.
Use struct sockaddr_in instead
struct sockaddr_in
{
u_short sin_family; /* type of address */
u_short sin_port; /* protocol port # */
u_long sin_addr; /* IP address */
char sin_zero[8]; /* filler (zeroed) */
};
IMPORTANT:
- ALWAYS USE NETWORK BYTE ORDER FOR THESE VALUES.
- socket()
- Creates a new socket. This needs to know the protocol family and the protocol type.
- connect()
- A client uses this to build a connection with a server.
- write()
- Send data through a connection.
- read()
- Receive data through a connection.
- close()
- Terminate a connection
- bind()
- Allows server to bind itself to a well known port
- listen()
- Changes the socket into a passive socket, waiting for new connections.
- accept()
- Wait for a new connection.
Client sequence of calls
- socket
- connect
- read, write
- close
Server sequence of calls (trivial server)
- socket
- bind
- listen
- accept
- read, write
- close
Server sequence of calls (forking)
- socket
- bind
- listen
- accept
- fork
- read, write
- close
- exit
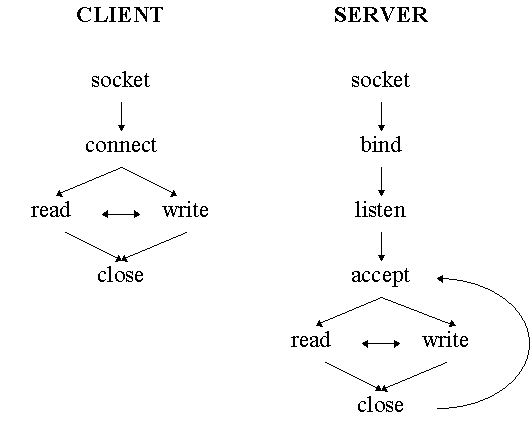