CS 596 Client-Server Programming
Intro Lecture
[To Lecture Notes Index]
San Diego State University -- This page last updated January 29, 1996

Contents of Intro Lecture
- Introduction to Course
- Introduction to Client-Server
- What is Client-Server?
- Theme Examples for Course
- What Client-Server Requires of a Programmer
- Comments
- Kinds of Comments
- Criteria for Evaluating for Modularity
- Computing "Paradigms"
- Centralized Multi-user Architecture
- Distributed Single-User Architecture
- Client/Server Architecture
Items To Cover
- Prerequisites
- Grades
- Programs
- Homework
- Projects
- Class notes, www
- Why two instructors
- How lectures will work
- Why this course
- When will it be offered again?
- Machines, accounts, languages
Client
-
- Application that initiates peer-to-peer communication
-
- Translate user requests into requests for data from server via protocol
-
- GUI often used to interact with user
Server
- Any program that waits for incoming communication requests from a client
-
- Extracts requested information from data and return to client
-
- Common Issues
- Authentication
- Authorization
- Data Security
- Privacy
- Protection
- Concurrency
Example: World Wide Web (WWW)
Data
-
- Server normally provides data to clients
-
- Often utilizes some data base
-
- WWW data is HyperText Markup Language (html) files
<!DOCTYPE HTML SYSTEM "html.dtd">
<HTML>
<HEAD><TITLE>
Client Server Programming
</TITLE></HEAD>
<BODY>
<H2>Client Server Programming</H2>
<HR>
Protocol
- How do the client and server interact
-
- This is the glue that make client-server work
-
- Involves using low level network protocols and application specific
protocols
-
- Designing application specific protocols is very important
-
- WWW uses the HyperText Transfer Protocol
Request | = | SimpleRequest | FullRequest |
SimpleRequest | = | GET <uri> CrLf |
FullRequest | = | Method URI ProtocolVersion CrLf |
| | [*<HTRQ Header>] |
| | [<CrLf> <data>] |
<Method> | = | <InitialAlpha> |
ProtocolVersion | = | HTTP/1.0 |
uri | = | <as defined in URL spec> |
<HTRQ Header> | = | <Fieldname> : <Value> <CrLf> |
<data> | = | MIME-conforming-message |
What this Course is not
An advanced (or beginning) Networking course
How to use a client builder application/system
- Powerbuilder
What this Course covers
The skills and knowledge required to build client-server applications
The Client-Server University (CSU) is building a Virtual Campus (VC) where
students and instructors interact via the Internet.
CSU needs some help in implementing programs to facilitate activities that
currently occur in person.
Posting Grades During the Semester
Faculty currently post grades on their office doors during the semester to let
students know how they are doing.
Submitting Final Grades to Registrar
Faculty currently bring signed formalized documents to the registrar with final
grades.
Office Hours
Students currently come to faculty offices.
Student Board Game Club
Students currently go to the student union to play chess, checkers and go
- Designing robust protocols
- Designing usable computer-human interfaces
- Good documentation skills
- Understand the information flow of the company/customer
- Multi-platform development
Nintendo
*
X := X + 1 /* add one to X
"Comments are easier to write poorly than well, and comments can be more
damaging than helpful"
X := X + 1 /* add one to X
/* if allocation flag is zero */
if ( AllocFlag == 0 ) ...
- Used to explain complicated or tricky code
*p++->*c = a
/* first we need to increase p by one, then ..
-
-
- Make code simpler before commenting
(*(p++))->*c = a
ObjectPointerPointer++;
ObjectPointer = *ObjectPointerPointer;
ObjectPointer ->*DataMemberPointer = a;
/* **** Need to add error checking here **** */
- Distills a few lines of code into one or two sentences
-
-
-
- Description of the code's intent
- Explains the purpose of a section of code
{ get current employee information } intent
{ update EmpRec structure } what
Programming
Progamming is a process that produces products
Products
- source code
- documentation
- design
- tools
Programming is a skill like writing or playing musical instrument
How does one develop a skill?
What is our metric to measure how well we program?
Decomposability
Decompose problem into smaller subproblems that can be solved separately
Example: Top-Down Design
Counter-example: Initialization Module
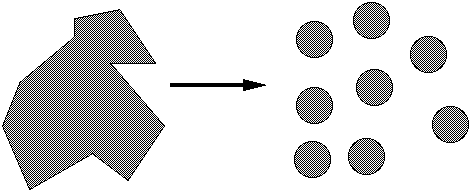
Criteria for Evaluating for Modularity
Composability
Freely combine modules to produce new systems
Examples: Math libraries
Unix command & pipes
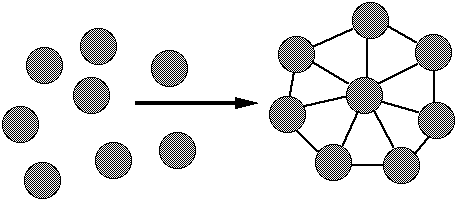
Criteria for Evaluating for Modularity
Understandability
Individual modules understandable by human reader
Counter-example: Sequential Dependencies
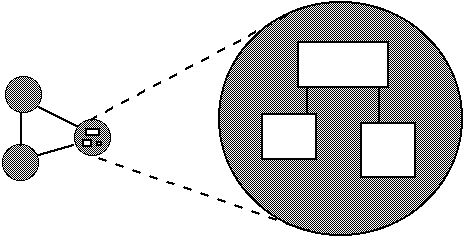
Criteria for Evaluating for Modularity
Continuity
Small change in specification results in:
- Changes in only a few modules
- Does not affect the architecture
Example: Symbolic Constants
const MaxSize = 100
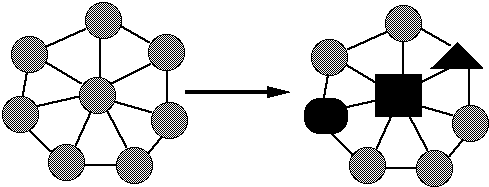
Criteria for Evaluating for Modularity
Protection
Effects of an abnormal run-time condition is confined to a few modules
Example: Validating input at source
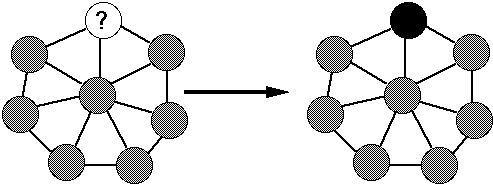
- Centralized Multi-user Architecture
- Distributed Single-User Architecture
- Client/Server Architecture
Large central computers serving many users
Motivating Factors
- Service large number of users (200 to 10,000+)
-
- Centralized storage for large data bases
-
- Minimize data on slow networks
Strengths
- Very stable, very reliable, well supported
-
- Cost-effective why to support thousands of users
-
- Large pool of technical staff
-
- Large number of business applications available
Weakness
- Proprietary hardware and software
-
- Very expensive
-
- Requires large support staff
-
- Costly to incrementally add more capacity
Mind Set
- Hierarchical organization (Bureaucratic heaven)
Motivating Factors
- Low cost fast local area networks
-
- Provide small number of users with compute power
-
- Failure of MIS departments to be responsive and cost-effective
Strengths
- Cheap hardware and software
-
- Lots of third-party software
-
- User is in complete control of environment
-
- Low cost to add more users
Weakness
- Sharing of resources across many users is difficult
-
- Networks and OS do not provide good control or management over computer
resources
-
- Multivender environments can cause operation, support and reliability
problems
Mind set
- Individualism (Lone Ranger syndrome)
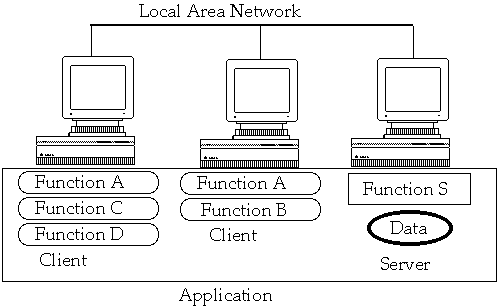
Motivating Factors
- Limitations of other modes of computing
-
- "Cool" applications like WWW
-
- Utilize easy to use micro computers as front end to mainframe computers
Strengths
-
- Cost-effective way to support thousands of users
-
- Low cost to add more users
-
- Cheap hardware and software
-
- Provides control over access to data
-
- User remains in control over local environment
-
- Flexible access to information
Weaknesses
- Reliability
-
- Complexity
-
- Lack of Maturity
-
- Lack of trained developers
-
- Politics
- A threat to the bureaucrats and the lone rangers