|
CS 635 Advanced Object-Oriented Design & Programming
Spring Semester, 2002
Assignment 1 Comments & Null Object
|
|
|
Previous   
Lecture Notes Index
   Next    
© 2002, All Rights Reserved, SDSU & Roger Whitney
San Diego State University -- This page last updated 05-Feb-02
|
|
Contents of Doc 3, Assignment 1 Comments & Null Object
References
Object-Oriented
Design Heuristics
,
Riel, Addison-Wesley, 1996,
Refactoring:
Improving the Design of Existing Code, Fowler, 1999, pp. 260-266
“Null
Object”, Woolf, in
Pattern
Languages of Program Design
3,
Edited by Martin, Riehle, Buschmmann, Addison-Wesley, 1998, pp. 5-18
NullObject
Structure
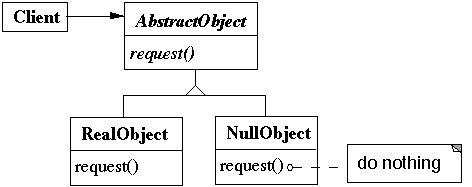
NullObject
implements all the operations of the real object,
These
operations do nothing or the correct thing for nothing
Binary
Search Tree Example
Without
Null Object
public class BinaryNode {
Node left = new NullNode();
Node right = new NullNode();
int key;
public boolean includes( int value ) {
if (key == value)
return true;
else if ((value < key) & left == null) )
return false;
else if (value < key)
return left.includes( value );
else if (right == null)
return false;
else
return right.includes(value);
}
etc.
}
Binary
Search Tree ExampleClass Structure
Object
Structure
Searching
for a Key
public class BinaryNode extends Node {
Node left = new NullNode();
Node right = new NullNode();
int key;
public boolean includes( int value ) {
if (key == value)
return true;
else if (value < key )
return left.includes( value );
else
return right.includes(value);
}
etc.
}
public class NullNode extends Node {
public boolean includes( int value ) {
return false;
}
etc.
}
Comments
on Example
- BinaryNode
always has two subtrees
- No
need check if left, right are null
- Since
NullNode has no state just need one instance
- Use
singleton pattern for the one instance
- Access
to NullNode is usually restricted to BinaryNode
-
- Forces
indicate that one may not want to use the Null Object pattern
- However,
familiarity with trees makes it easy to explain the pattern
- Implementing
an add method in NullNode
-
- Requires
reference to parent or
- Use
proxy
-
Refactoring
Introduce
Null Object[2]
You
have repeated checks for a null value
Replace
the null value with a null object
Example
customer isNil
ifTrue: [plan := BillingPlan basic]
ifFalse: [plan := customer plan]
becomes:
- Create
NullCustomer subclass of Customer with:
NullCustomer>>plan
^BillingPlan basic
- Make
sure that each customer variable has either a real customer or a NullCustomer
Now
the code is:
plan := customer plan
- Often
one makes a Null Object a singleton
Applicability
Use
the Null Object pattern when:
- Some
collaborator instances should do nothing
- You
want clients to ignore the difference between a collaborator that does
something and one that does nothing
- Client
does not have to explicitly check for null or some other special value
- You
want to be able to reuse the do-nothing behavior so that various clients that
need this behavior will consistently work in the same way
Use
a variable containing null or some other special value instead of the Null
Object pattern when:
- Very
little code actually uses the variable directly
- The
code that does use the variable is well encapsulated - at least in one class
- The
code that uses the variable can easily decide how to handle the null case and
will always handle it the same way
Consequences
Advantages
- Encapsulates
do nothing behavior
- Makes
do nothing behavior reusable
Disadvantages
-
- Makes
it difficult to distribute or mix into the behavior of several collaborating
objects
- May
cause class explosion
-
- Different
clients may have different idea of what “do nothing” means
-
- NullObject
objects can not transform themselves into a RealObject
Implementation
Too
Many classes
- Eliminate
one class by making NullObject a subclass of RealObject
Multiple
Do-nothing meanings
-
- If
different clients expect do nothing to mean different things use Adapter
pattern to provide different do-nothing behavior to NullObject
Transformation
to RealObject
- In
some cases a message to NullObject should transform it to a real object
- Use
the proxy pattern
[1]
See Riel
[2]
Refactoring Text, pp. 260-266
Copyright ©, All rights reserved.
2002 SDSU & Roger Whitney, 5500 Campanile Drive, San Diego, CA 92182-7700 USA.
OpenContent license defines the copyright on this document.
Previous   
visitors since 05-Feb-02
   Next