 |
CS 696 Emerging Technologies: Distributed Objects |
|
---|
Spring Semester, 1998
CORBA Intro
To Lecture Notes Index
© 1998, All Rights Reserved, SDSU & Roger Whitney
San Diego State University -- This page last updated 21-Apr-98
Contents of Doc 19, CORBA Intro
- References
- Introduction
- CORBA Architecture
- The Broker Pattern
- Dynamics
- Variants
- Known Uses
- Consequences
- A Simple CORBA Example using OrbixWeb
Pattern-Oriented Software: A System of Patterns, Buschman, Meunier,
Rohnert, Sommerlad, Stal, 1996, pp 99-122 (Broker pattern)
OMG web site: http://www.omg.org
OrbixWeb Programmer's Guide, IONA Technologies PLC, November 1997,
chapter 2 Getting Started with Java Applications, pp 33 - 54
Inside CORBA: Distributed Object Standards and Applications, Mowbray
& Ruh, Addison-Wesley, 1997
CORBA - Common Object Request Broker Architecture
OMG
- Object Management Group
-
- Develops CORBA standards, but does not implement
- See: http://www.omg.org for more
information on OMG and CORBA
Some ORB Venders
Iona - Orbix, OrbixWeb
Visigenic - Visibroker
Sun - Java IDL in JDK 1.2
Object Oriented Concepts - OmniBroker
Object Space - Voyager
A list of ORB, free and otherwise can be found at:
- http://adams.patriot.net/~tvalesky/freecorba.html
Some History
Event | Date |
OMG Formed | 1989 |
CORBA 1 | 1991 |
CORBA 1.2 | June, 1994 |
Smalltalk Language Mapping | March 28, 1995 |
Time Service | March 19, 1996 |
C++ Language Mapping 1.1 | March 19, 1996 |
Ada Language Mapping | March 19, 1996 |
CORBA 2.0 | July 1, 1996 |
Object Trader Service | October 30, 1996 |
CORBA Interoperability | October 30, 1996 |
IDL COBOL Mapping | March 11, 1997 |
Transaction Service 1.1 | June 24, 1997 |
IDL Java Mapping 1.0 | June 24, 1997 |
CORBA 2.1 | September 23, 1997 |
COM/CORBA Part B | November 19, 1997 |
COM/CORBA Part A Revision | November 19, 1997 |
Mobile Agents Facility | February 10, 1998 |
CORBA 2.2 | March 2, 1998 |
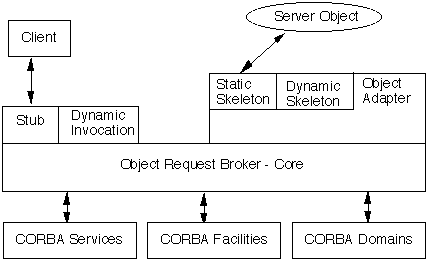
Object Request Broker
- Provides an infrastructure allowing objects to interact, independent of the
specific platforms and languages used to implement the objects.
Object Services
- Standardize the life-cycle management of objects
-
- Services cover such items as
- Creating objects
- Controlling access to objects
- Keeping track of relocated objects,
- Controlling the relationship between styles of objects
- Object Services provide for application consistency
Common Facilities
- Commercially known as CORBAfacilities
-
- A set of generic application functions such as
- printing
- document management
- database
- electronic mail facilities
- Include facilities for use over the Internet
Domain Interfaces
- Vertical areas that provide functionality of direct interest to end-users
in particular application domains
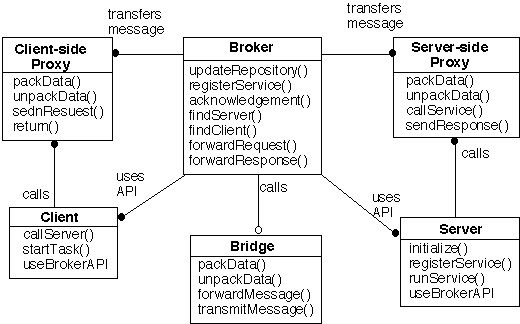
A broker
- Handles the transmission of requests from clients to servers
-
- Handles the transmission of responses from servers to clients
-
- Must have some means to identify and locate server
-
- If server is hosted by different broker, forwards the request to other
broker
-
- If server is inactive, active the server
-
- Provides APIs for registering servers and invoking server methods
Bridge
- Optional components used for hiding implementation details when two brokers
interoperate
Registering Server
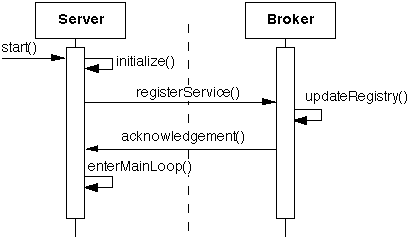
Client Server Interaction
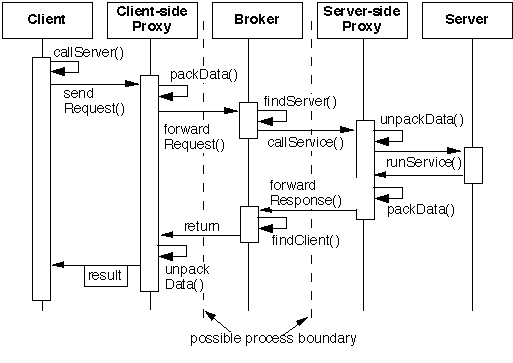
Direct Communication Broker System
- Broker gives the client a communication channel to the server
-
- Client and server interact directly
-
- Many CORBA implementation use this variant
Message Passing Broker System
- Clients and servers pass messages rather than services (methods)
Trader System
- Clients request a service, not a server
-
- Broker forwards the request to a server that provides the service
Adapter Broker System
- Hide the interface of the broker component to the servers using an
additional layer
-
- The adapter layer is responsible for registering servers and interacting
with servers
- For example if all server objects are on the same machine as application a
special adapter could link the objects to the application directly
Callback Broker System
- Eliminate the difference between clients and servers
- When an event is registered with a broker, it calls the component that is
registered to handle the event
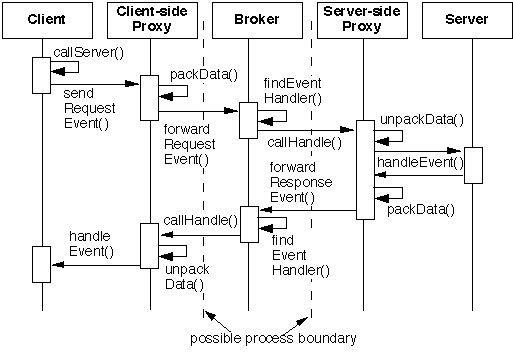
CORBA
IBM's SOM/DSOM
Mircosoft OLE 2.x
RMI
Benefits
Location Transparency
- Clients (servers) do not care where servers (clients)are located
Changeability and extensibility of components
- Changes to server implementations are transparent to clients if they don't
change interfaces
-
- Changes to internal broker implementation does not affect clients and
servers
-
- One can change communication mechanisms without changing client and server
code
Portability of Broker System
- Porting client & servers to a new system usually just requires
recompiling the code
Benefits - Continued
Interoperability between different Broker System
- Different broker systems may interoperate if they have a common protocol
for the exchange of messages
-
- CORBA defines a general inter-ORB protocol (GIOP) for orb to orb
communication
- Internet inter--ORB protocol (IIOP) is the TCP/IP version of GIOP
-
- DCOM and CORBA interoperate
-
- DCOM and RMI interoperate
-
- Soon RMI and CORBA will interoperate
Reusability
- In building new clients you can reuse existing services
Liabilities
Restricted Efficiency
Lower fault tolerance compared to non-distributed software
Benefits and Liabilities
Testing and Debugging
- A client application using tested services is easier to test than creating
the software from scratch
-
- Debugging a Broker system can be difficult
What is an Object Request Broker (ORB)?
Step 1 Create an interface for the server using the CORBA interface
definition language (IDL)
Place the following code in the file Hello.idl
interface Hello
{
string sayHello();
};
Step 2 Compile the IDL interface
idl Hello.idl
This creates a directory called java_output which contains:
Hello.java | HelloPackage/ | _HelloSkeleton.java |
HelloHelper.java | _HelloImplBase.java | _HelloStub.java |
HelloHolder.java | _HelloOperations.java | _tie_Hello.java |
IDL output
Hello
- Client interface to server
_HelloStub
- Client side proxy for server
_HelloSkeleton
- Server side proxy
_HelloImplBase
- Abstract class to use as parent class to server
-
- This is called the ImplBase approach
_tie_Hello
- Java class which allows server to use composition (delegation) rather than
having to inherit from _HelloImplBase
- This is called the TIE approach
_HelloOperations
- Used in the TIE approach
-
- Java interface that maps the attributes and operations of the IDL
definition to Java methods
HelloPackage
- A Java package used to contain any IDL types nested in the Hello
interface
HelloHelper
- A Java class that allows IDL user-defined types to be manipulated in
various ways
HelloHolder
- Used for passing Hello objects as parameters
Step 3 Implementing the Server using Inheritance
Classes generated by OrbixWeb IDL compiler Hello
public interface Hello
extends org.omg.CORBA.Object
{
public String sayHello() ;
public java.lang.Object _deref() ;
}
_HelloImplBase
import IE.Iona.OrbixWeb._OrbixWeb;
public abstract class _HelloImplBase
extends _HelloSkeleton
implements Hello
{
public _HelloImplBase() {
org.omg.CORBA.ORB.init().connect(this);
}
public _HelloImplBase(String marker) {
_OrbixWeb.ORB(org.omg.CORBA.ORB.init()).connect(this,marker);
}
public _HelloImplBase(IE.Iona.OrbixWeb.Features.LoaderClass loader) {
_OrbixWeb.ORB(org.omg.CORBA.ORB.init()).connect(this,loader);
}
public _HelloImplBase(String marker,
IE.Iona.OrbixWeb.Features.LoaderClass loader) {
_OrbixWeb.ORB(org.omg.CORBA.ORB.init()).connect(this,marker,loader);
}
public java.lang.Object _deref() {
return this;
}
}
Programmer Implemented ClassesHelloImplementation
public class HelloImplementation extends _HelloImplBase
{
public String sayHello()
{
return "Hello World";
}
}
HelloServer
import IE.Iona.OrbixWeb._CORBA;
import IE.Iona.OrbixWeb.CORBA.ORB;
public class HelloServer
{
public static void main (String args[])
{
org.omg.CORBA.ORB ord =
org.omg.CORBA.ORB.init();
try
{
Hello server = new HelloImplementation();
_CORBA.Orbix.impl_is_ready( "HelloServer" );
System.out.println("Server going Down");
}
catch ( org.omg.CORBA.SystemException corbaError)
{
System.out.println("Exception " + corbaError);
}
}
}
Step 4 Compiling the Server
The classpath must include the following:
- Java JDK classes
- org.omg.CORBA package
- IR.Iona.OrbixWeb package
Must compile the following classes:
Hello.java
_HelloSkeleton.java
_HelloImplBase.java
HelloImplementation.java
HelloServer.java
Step 5 Registering and running the Server
Make sure that the OrbixWeb daemon (orbixdj) is running on the server
machine
You start the deamon by the command:
orbixdj -textConsole
Now register the server via:
putit HelloServer -java HelloServer
Now run the server via
java HelloServer
Note running the server is not normally required, however, since the server is
not in a package it is hard to get the ORB to activate the server. We will
address this issue later
Details of the above process will be discussed later
Step 6 Writing the client
HelloClient.java
import IE.Iona.OrbixWeb._CORBA;
import org.omg.CORBA.ORB;
public class HelloClient
{
public static void main(String args[])
{
ORB.init();
String hostname = "eli.sdsu.edu";
String serverLabel = ":HelloServer";
Hello server = HelloHelper.bind( serverLabel, hostname);
System.out.println( server.sayHello() );
}
}
Step 7 Compiling and Running the client
Compile the classes:
_HelloStub.java
HelloClient.java
Now run the client with the command:
java HelloClient
Output - Client Window
[New IIOP Connection (eli.sdsu.edu,IT_daemon, null,null,pid=0) ]
[New IIOP Connection (eli.sdsu.edu,HelloServer, null,null,pid=0) ]
Hello World
Output - Server Window
[ HelloServer: New Connection (eli.sdsu.edu:59201) ]
[ HelloServer: End of IIOP connection (eli.sdsu.edu:59201) ]
Output - Daemon Window
[ IT_daemon: New Connection (eli.sdsu.edu:59200) ]
[ IT_daemon: End of IIOP connection (eli.sdsu.edu:59200) ]
visitors since 17-Mar-98